Calibrating volatility smiles with SABR
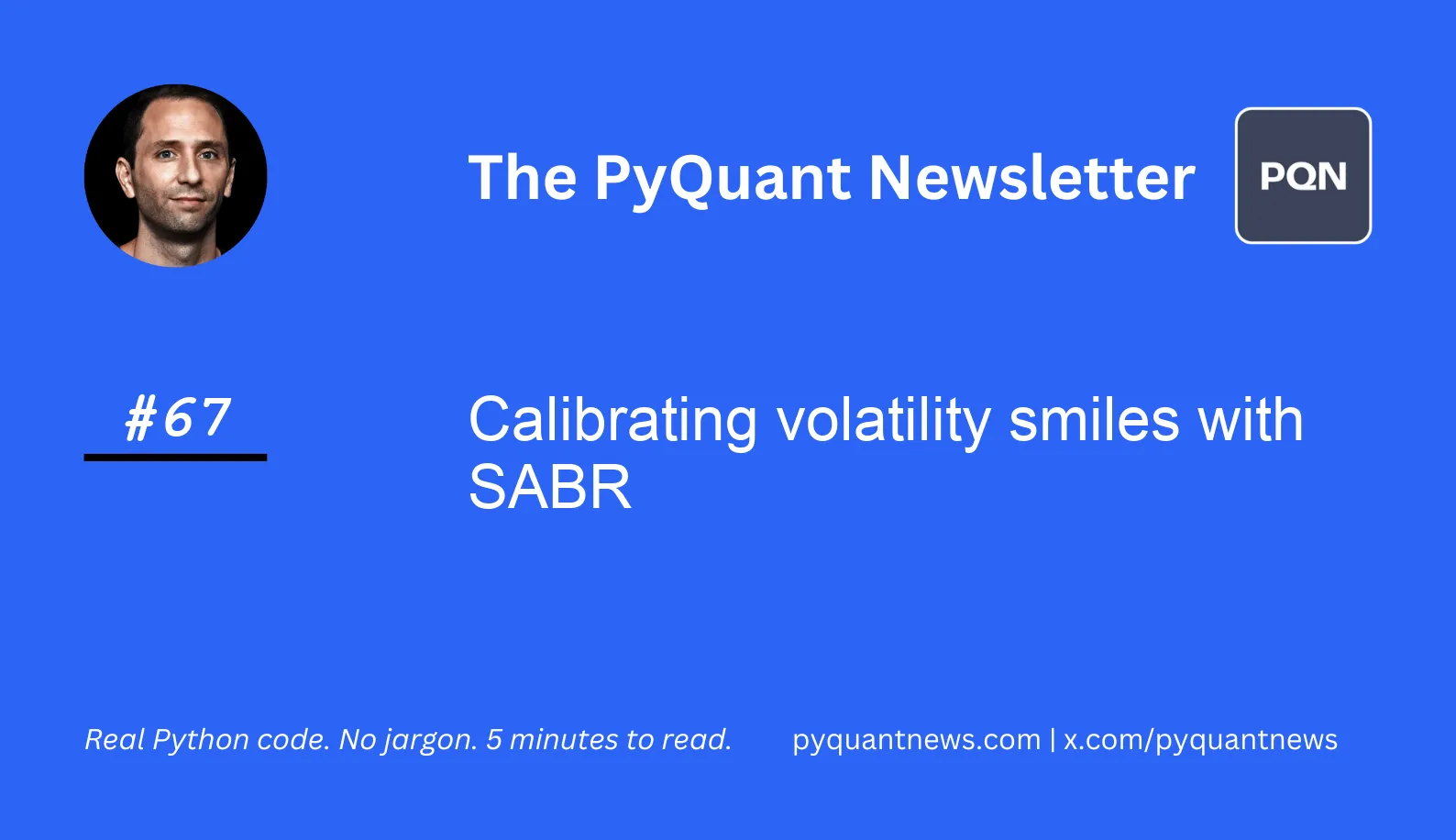
Calibrating volatility smiles with SABR
In today's newsletter, we’ll explore the SABR stochastic volatility model.
It’s a very popular volatility model used by professionals for many types of derivatives.
Today, we’ll look at how to calibrate the SABR parameters and use them to fit a volatility smile for equity options.
Sound good?
Let’s go!
Calibrating volatility smiles with SABR
The SABR model, built in 2002, stands as a key stochastic volatility framework in finance for modeling derivatives. SABR stands for "stochastic alpha, beta, rho" which are the key inputs to the model.
SABR is good at capturing the market-observed anomalies such as skew and smile.
It operates on the premise that asset prices follow a geometric Brownian motion while volatility follows an Ornstein-Uhlenbeck process, parameterized by alpha (initial volatility level), beta (variance elasticity), rho (price-volatility correlation), and nu (volatility's volatility).
One of its standout features is the closed-form approximation for implied volatility—known as the Hagan formula—which allows for fast and accurate computations of implied volatility across strike prices.
Ready?!
Imports and set up
We’ll use the pysabr library to calibrate the SABR model, fit the volatility smile, and price options. Let’s import the libraries.
1import pandas as pd
2import numpy as np
3import matplotlib.pyplot as plt
4from openbb_terminal.sdk import openbb
5from pysabr import Hagan2002LognormalSABR
6from pysabr import hagan_2002_lognormal_sabr as sabr
7from pysabr.black import lognormal_call
We’ll use OpenBB to fetch options prices for a ticker.
1symbol = "SPY"
2expiration = "2026-01-16"
3spy = openbb.stocks.options.chains(symbol, source="YahooFinance")
From here, we’ll grab the calls and puts at the specified expiration.
1calls = spy[spy.optionType == "call"]
2jan_2026_c = calls[calls.expiration == expiration].set_index("strike")
3jan_2026_c["mid"] = (jan_2026_c.bid + jan_2026_c.ask) / 2
4
5puts = spy[spy.optionType == "put"]
6jan_2026_p = puts[puts.expiration == expiration].set_index("strike")
7jan_2026_p["mid"] = (jan_2026_p.bid + jan_2026_p.ask) / 2
8
9strikes = jan_2026_c.index
10vols = jan_2026_c.impliedVolatility * 100
We filter the DataFrame for call and put options at the given expiration. We then compute the mid price to get a more accurate picture of implied volatility. We’ll need the strikes and market implied volatilities, too.
Fit the SABR model
First, we compute the forward stock price using put-call parity, set the expiration, and assign a beta.
1f = (
2 (jan_2026_c.mid - jan_2026_p.mid)
3 .dropna()
4 .abs()
5 .sort_values()
6 .index[0]
7)
8t = (pd.Timestamp(expiration) - pd.Timestamp.now()).days / 365
9beta = 0.5
Put-call parity defines the relationship between put options, call options, and the forward price of the underlying. You can read more here. The t parameter is the fraction of time until expiration.
The beta parameter governs the shape of the forward rate, which influences the shape of the implied volatility smile. A beta of 1 implies a lognormal distribution, which is consistent with the Black-Scholes model. Moving beta away from 1 allows for different shapes of volatility smiles. In practice, setting beta to 0.5 is usually a safe bet.
Once we set the parameters, we instantiate the model and call fit to get alpha, rho, and volvol.
1sabr_lognormal = Hagan2002LognormalSABR(
2 f=f,
3 t=t,
4 beta=beta
5)
6
7alpha, rho, volvol = sabr_lognormal.fit(strikes, vols)
The fit method calibrates the SABR model parameters to best fit a given volatility smile. The alpha parameter is a measure of the at-the-money volatility level, rho represents the correlation between the asset price and its volatility, and volvol which is the volatility of volatility.
Generate a fitted volatility smile
Now that we have alpha, rho, and volvol, we can generate the calibrated volatility smile.
1calibrated_vols = [
2 sabr.lognormal_vol(strike, f, t, alpha, beta, rho, volvol) * 100
3 for strike in strikes
4]
This code generates a lognormal volatility for each strike in our options chain.
Let’s plot it.
1plt.plot(
2 strikes,
3 calibrated_vols
4)
5
6plt.xlabel("Strike")
7plt.ylabel("Volatility")
8plt.title("Volatility Smile")
9plt.plot(strikes, vols)
10plt.show()
The result is a fitted volatility smile (or smirk in this case), along with the market based volatility smile.
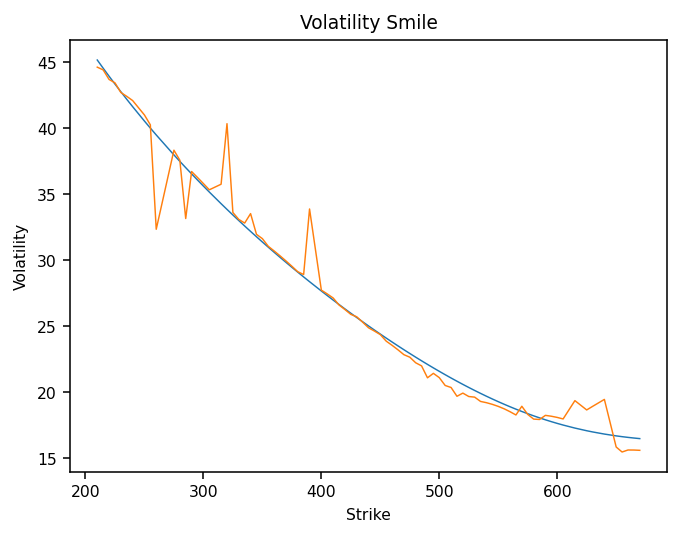
We can assess the model error as well.
1black_values = []
2for strike, calibrated_vol in zip(strikes.tolist(), calibrated_vols):
3 black_value = lognormal_call(
4 strike,
5 f,
6 t,
7 calibrated_vol / 100,
8 0.05,
9 cp="call"
10 )
11 black_values.append(black_value)
12
13option_values = pd.DataFrame(
14 {
15 "black": black_values,
16 "market": jan_2026_c.mid
17 },
18 index=strikes
19)
20
21(option_values.black - option_values.market).plot.bar()
The result is a bar chart which demonstrates the model error for each strike price based on the calibrated implied volatility from the SABR model.
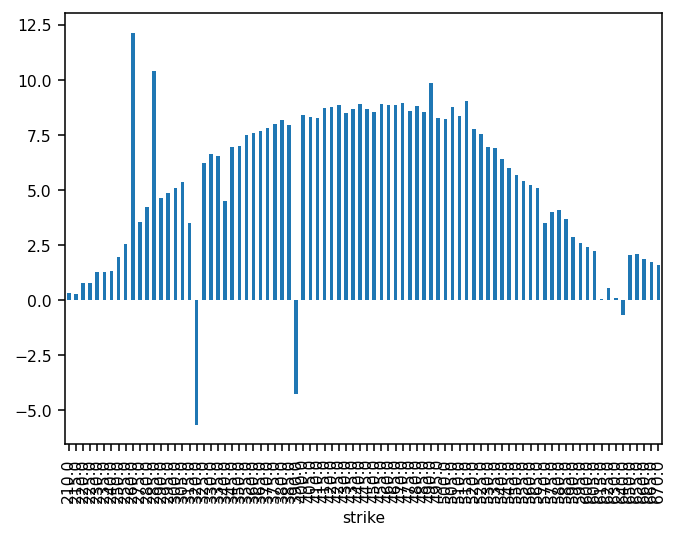
Next steps
We breezed over a lot of the theory behind the SABR model. As a next step, take a look at the Wikipedia article on the SABR model. It will help get more intuition behind what’s happening.
