Use expectancy to boost your winning trades
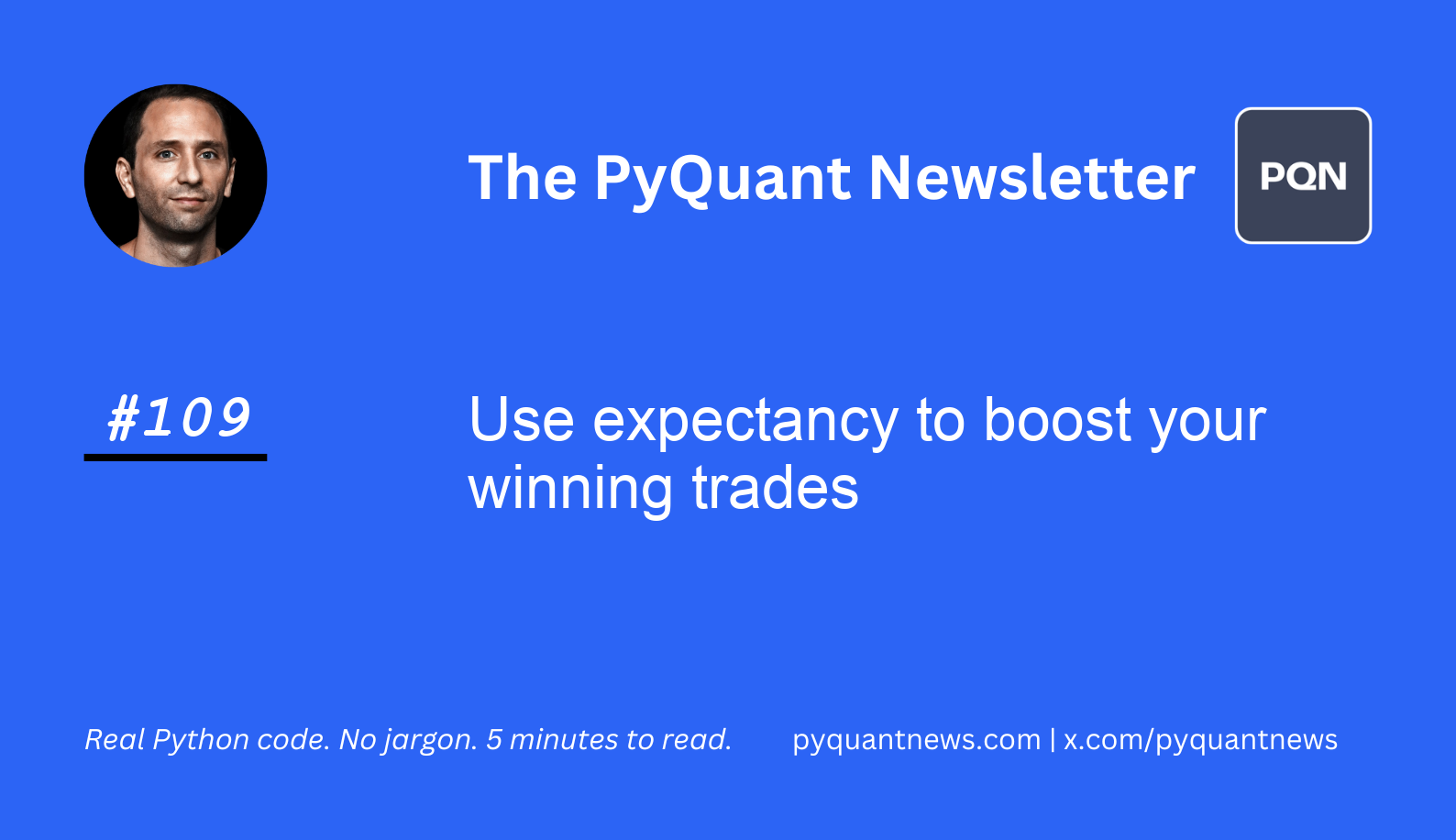
Use expectancy to boost your winning trades
Trading profitably comes down to one thing:
Edge.
Most people have heard of edge but don’t actually know what it is. Edge refers to positive expected value in a trade. It’s the probability-wighted value of all payoffs summed over all possible outcomes.
Traders use the concept of expectancy as a proxy for expected value in trading.
It’s a critical concept to understand and took me years to finally get my head around it. But once I did, I was able to focus on strategies that had a positive expected value. By reading today’s newsletter, you’ll get a simple Python function to compute expectancy.
Let’s go!
Use expectancy to boost your winning trades
Expectancy in algorithmic trading seeks to predict the average outcome of trades. It combines probabilities of winning and losing with average profit and loss. This helps traders understand potential gains or losses per trade.
And of course measure their edge.
Traders calculate expectancy using a formula involving probabilities and averages. A positive expectancy indicates a profitable strategy, while a negative one suggests losses. Applying this formula involves gathering historical data, calculating probabilities, and determining average wins and losses.
Professional traders use expectancy for risk management, strategy evaluation, and capital allocation. They set stop-loss and take-profit levels, compare strategies, and determine optimal position sizes. It helps in making informed decisions and improving trading performance.
Let’s see how it works with Python.
Import necessary libraries to compute expectancy ratio
We will start by importing the necessary libraries to handle data and perform calculations.
1import pandas as pd
2import numpy as np
Pandas will help us manage and manipulate our trading data, while NumPy will assist with numerical calculations.
Define function to calculate expectancy ratio
Next, we will define a function that takes a DataFrame of trade results and calculates the expectancy ratio.
1def calculate_expectancy_ratio(trades):
2 num_trades = len(trades)
3 winners = trades[trades['Profit'] > 0]
4 losers = trades[trades['Profit'] <= 0]
5
6 win_rate = len(winners) / num_trades
7 loss_rate = len(losers) / num_trades
8
9 avg_win = winners['Profit'].mean()
10 avg_loss = losers['Profit'].mean()
11
12 expectancy_ratio = (win_rate * avg_win) + (loss_rate * avg_loss)
13
14 return expectancy_ratio
This function calculates the expectancy ratio by first determining the number of trades, winners, and losers. It then calculates the win rate and loss rate. The average profit of winning trades and the average loss of losing trades are computed. Finally, the expectancy ratio is calculated using these values.
Create a sample DataFrame of trade results and compute expectancy ratio
We will create a sample DataFrame to simulate trade results and then use our function to compute the expectancy ratio.
1trade_data = {
2 'Trade': [1, 2, 3, 4, 5, 6, 7, 8, 9, 10],
3 'Profit': [100, -50, 200, -100, 300, -150, 400, -200, 500, -250]
4}
5
6trades = pd.DataFrame(trade_data)
7
8expectancy_ratio = calculate_expectancy_ratio(trades)
9print(f"Expectancy Ratio: {expectancy_ratio}")
We create a dictionary with trade numbers and corresponding profit/loss values. This dictionary is then converted into a DataFrame. We then call the calculate_expectancy_ratio
function, passing our DataFrame as an argument. The resulting expectancy ratio is printed to the screen.
Your next steps
This is a simple example. As a next step, replace the example trade results with your own results. Using pandas, you can import this from a CSV or manually create it. Most backtest engines will output a list of PnL as well.
