The easy way to detect important trading signals with Gamma
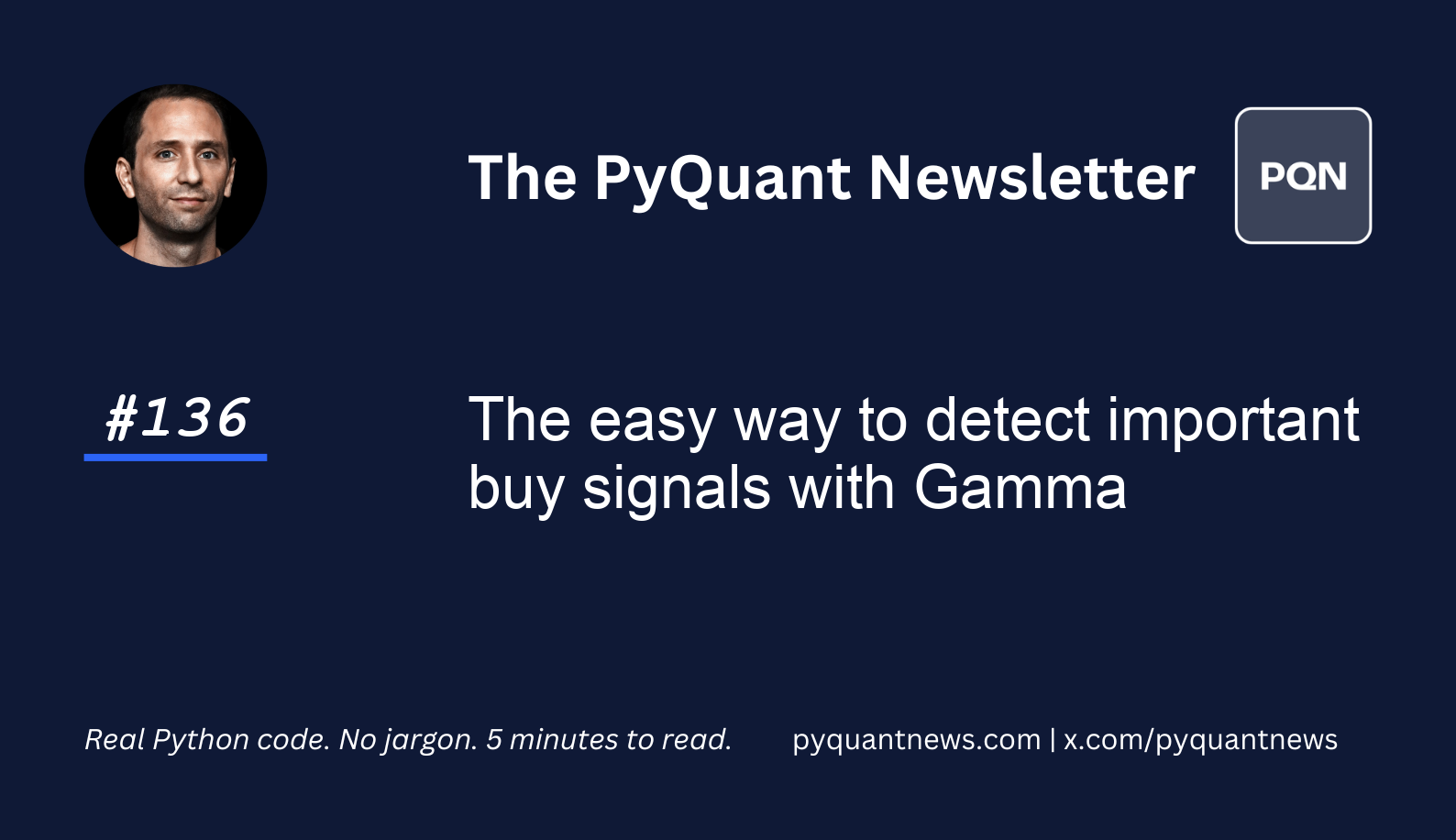
The easy way to detect important trading signals with Gamma
Last week, I introduced you to my friends at MenthorQ.
MenthorQ uses the options market to generate trading signals for stocks and futures.
I got a bunch of emails asking to give some details on how investors can use Gamma levels in their stock trading.
Today’s newsletter walks through collecting options data, computing Gamma, and visualizing it to find interesting stock levels.
Let's go!
The easy way to detect important trading signals with Gamma
In options trading, understanding gamma is key to identifying profitable buy and sell levels, especially as expiration dates approach. Gamma influences how delta, another option Greek, changes with stock price movements, offering valuable insights for traders nearing expiration.
In practice, gamma's effect becomes pronounced near expiration, revealing opportunities in volatile markets. Traders observe delta shifts, where gamma highlights significant movements. This insight aids in predicting price fluctuations, allowing traders to establish optimal buy or sell points during key events.
Professionals leverage gamma through strategies like gamma scalping, which involves frequent portfolio adjustments as delta alters. They use gamma indicators to anticipate option price volatility, positioning trades advantageously. Additionally, professionals utilize gamma-informed risk management techniques to navigate market-induced risks, mitigating potential losses.
Let's see how it works with Python.
Imports and set up
Yahoo recently broke the yFinance library so make sure to upgrade. You can use this command in your Jupyter Notebook.
1!pip install yfinance --upgrade --no-cache-dir
These libraries provide tools for data manipulation, numerical operations, visualization, and financial data retrieval.
1import pandas as pd
2import numpy as np
3import matplotlib.pyplot as plt
4from scipy.stats import norm
5import yfinance as yf
Fetch data for options and the underlying
We start by fetching option data for a specific ticker and expiration date using the yfinance library.
1ticker = "QQQ"
2expiration_date = "2025-02-28"
3
4stock = yf.Ticker(ticker)
5options = stock.option_chain(expiration_date)
6calls = options.calls
We use yfinance to get option data for the QQQ ticker with an expiration date of February 28, 2025. We retrieve the full option chain and then isolate the call options. This gives us a dataset of call options to work with for our analysis.
Build a function to compute Gamma and apply it to the options chains
Next, we define a function to calculate the gamma of a call option and apply it to our dataset.
1def call_gamma(S, K, T, r, sigma):
2 d1 = (np.log(S / K) + (r + 0.5 * sigma ** 2) * T) / (sigma * np.sqrt(T))
3 return norm.pdf(d1) / (S * sigma * np.sqrt(T))
4
5
6# Use navPrice for ETFs or currentPrice for for stocks
7S = stock.info["navPrice"]
8r = 0.0423 # Risk-free rate
9T = ((pd.to_datetime(expiration_date) - pd.Timestamp.now()).days + 1) / 365
10
11atm_strike = (
12 calls
13 .strike[calls.strike >= S]
14 .sort_values()
15 .iloc[0]
16)
17
18calls['gamma'] = (
19 calls
20 .apply(lambda row: call_gamma(S, row['strike'], T, r, row['impliedVolatility']), axis=1)
21)
We define a function to calculate the gamma of a call option using the Black-Scholes formula. We then set up our parameters, including the current price, risk-free rate, and time to expiration. We find the at-the-money strike price and calculate the gamma for each call option in our dataset.
Isolate open interest and Gamma and visualize the levels
We filter our data to focus on options near the current price and select the relevant columns for our analysis.
1gamma = (
2 calls[
3 (calls.strike >= atm_strike * 0.95) & (calls.strike <= atm_strike * 1.05)
4 ][[
5 "strike",
6 "impliedVolatility",
7 "openInterest",
8 "gamma"
9 ]]
10)
We create a new DataFrame called 'gamma' that contains options with strike prices within 5% of the at-the-money strike. We select the strike price, implied volatility, open interest, and calculated gamma for each of these options. This gives us a focused dataset for our visualization.
Finally, we create a plot to visualize the gamma distribution and open interest for our selected options.
1fig, ax1 = plt.subplots(figsize=(12, 6))
2
3ax1.plot(gamma['strike'], gamma['gamma'], label='Total Gamma', color='b')
4ax1.axvline(S, color='r', linestyle='--', label='Current Price')
5ax1.set_xlabel('Strike Price')
6ax1.set_ylabel('Gamma', color='b')
7ax1.tick_params(axis='y', labelcolor='b')
8
9ax1.set_ylim(0.00, ax1.get_ylim()[1])
10
11ax2 = ax1.twinx()
12ax2.bar(gamma['strike'], gamma['openInterest'], alpha=0.3, color='gray', label='Open Interest')
13ax2.set_ylabel('Open Interest', color='gray')
14ax2.tick_params(axis='y', labelcolor='gray')
15
16plt.title(f'Gamma Distribution for {ticker} Options')
17fig.legend(loc="upper left", bbox_to_anchor=(0.1,0.9))
18ax1.grid(True)
19
20plt.show()
We create a plot with two y-axes. The primary axis shows the gamma distribution as a blue line, with a red vertical line indicating the current underlying price.
The result is an image that looks like this.
.png)
Where you you see strikes with relatively
The peak gamma levels (blue line) suggest potential areas of high dealer hedging activity. Coupled with high open interest (gray bar), the levels can act as support and resistance zones where price volatility might increase or stabilize.
For example, we see a high level of open interest at the 540 strike price. This would be an area to watch for if long QQQ.
Your next steps
Try different underlying symbols and different expiration dates. You can also try to apply the same analysis for put options across different expirations.
