Build a powerful AI finance agent with Python
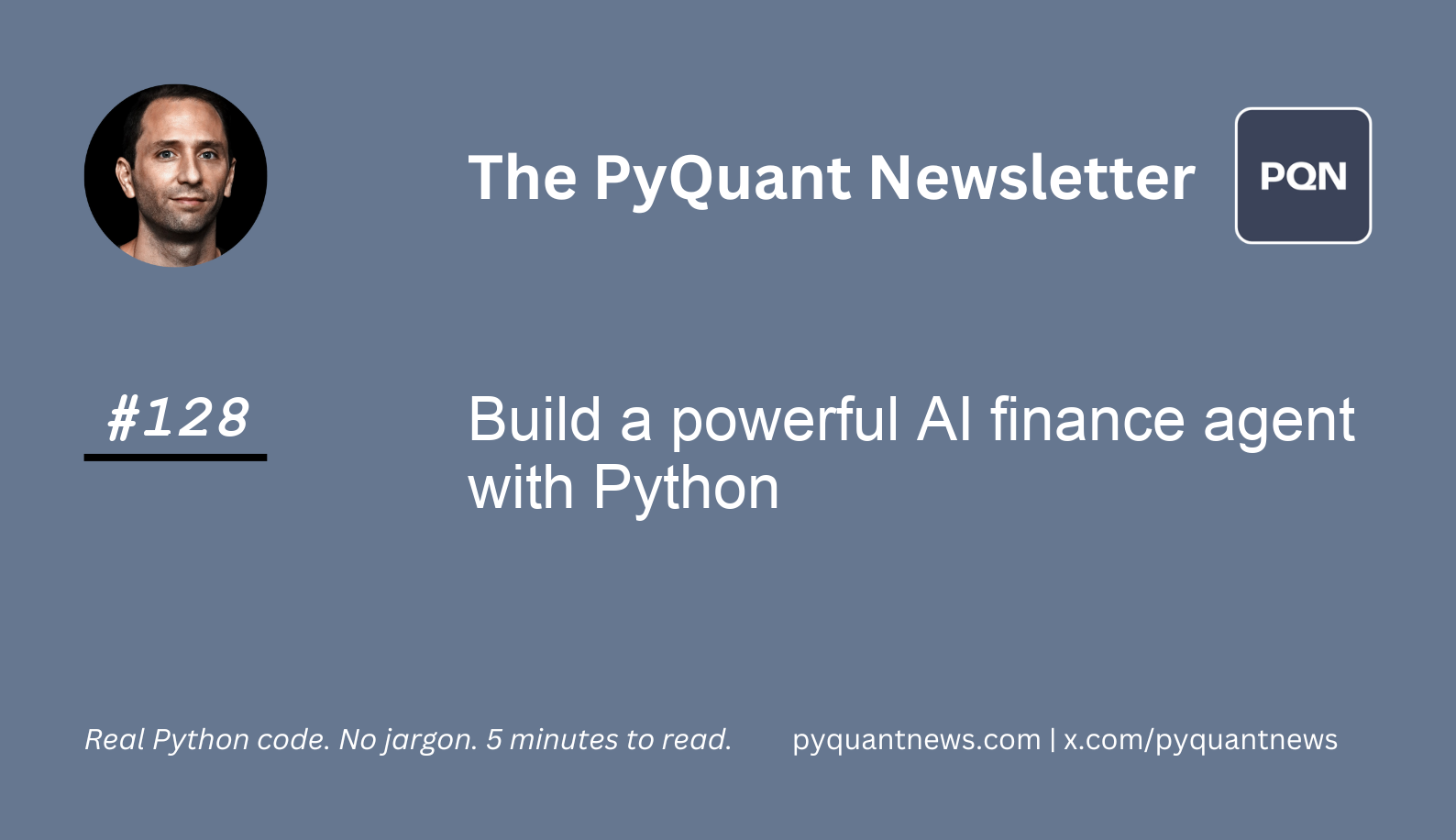
Build a powerful AI finance agent with Python
We already know that AI can read financial statements.
What about analyzing stock price data?
You may have heard about AI agents and agentic workflows. Agents are actually just a series of steps put together to accomplish a specific goal. These steps are stitched together with special prompts that let the AI reason about what to do.
Agents are the future of AI and are powerful ways to speed up your data processing workflow.
By reading today’s newsletter, you’ll get Python code to build an AI agent to write its own Python code to analyze stock price data.
Let’s go!
Build a powerful AI finance agent with Python
Python is changing how we integrate LLMs into market analysis workflows.
Analysts used to spend countless hours combing market data. Then, they'd spend more time manually manipulating this data. Now, AI can process and index data by itself.
When integrated with LLMs, Python can bring new levels of power and scalability to our analysis.
That's where LlamaIndex comes in.
Many companies and research organizations use LlamaIndex to streamline data workflows using LLMs.
LlamaIndex is designed for building, managing, and querying LLMs using external data. It offers a flexible ecosystem for integrating LLMs into applications. By handling the heavy lifting of data indexing, retrieval, and query processing, LlamaIndex helps developers focus on analysis instead of infrastructure.
Let’s see how it works.
Imports and set up
We start by loading the libraries and tools to set up our environment. This includes loading our Claude API key which is stored in a .env file.
1from llama_index.llms.anthropic import Anthropic
2from llama_index.core import Settings
3from llama_index.tools.code_interpreter.base import CodeInterpreterToolSpec
4from llama_index.core.agent import FunctionCallingAgent
5from dotenv import load_dotenv
6load_dotenv()
7
8
9code_spec = CodeInterpreterToolSpec()
10tools = code_spec.to_tool_list()
After importing the modules, we initialize a CodeInterpreterToolSpec
to translate its specifications into a list of tools, which are then stored in the variable tools
.
Configure the LLM with Anthropic’s Claude Sonnet
Next, we configure the language model and set it up to work with tokenization.
1tokenizer = Anthropic().tokenizer
2Settings.tokenizer = tokenizer
3
4llm_claude = Anthropic(model="claude-3-5-sonnet-20241022")
The code initializes the tokenizer from an Anthropic language model instance. Tokenizers break down text into a format that models can understand.
We update the global settings to use this tokenizer, ensuring consistency in text processing. We then create an instance of the language model claude-3-5-sonnet-20241022
, which will be used for generating and interpreting natural language responses.
Set up an agent to interact with the model
Here, we create an agent that will facilitate communication between our tools and the language model.
1agent = FunctionCallingAgent.from_tools(
2 tools,
3 llm=llm_claude,
4 verbose=True,
5 allow_parallel_tool_calls=False,
6)
The FunctionCallingAgent
is constructed using the tools defined earlier and the initialized language model. We pass verbose=True
to enable detailed logging of the agent's operations, which helps in debugging and understanding its actions.
By setting allow_parallel_tool_calls=False
, we ensure that the tools are called sequentially, which can prevent conflicts and ensure reliable execution.
This agent will handle the interaction between our code and the language model.
Write a prompt to analyze stock data
Finally, we draft a prompt that instructs the language model to generate Python code. This code will fetch and analyze stock data for a specified company over the past year.
1ticker = "PLTR"
2
3prompt = f"""
4Write Python code to:
5- Detect which date is today
6- Based on this date, fetch historical prices of {ticker} from the beginning of the month until today.
7- Analyze the last month prices
8"""
9
10resp = agent.chat(prompt)
We define a ticker
symbol representing the stock we want to analyze. The prompt is a formatted string that instructs the language model to produce Python code. This code should determine the current date, retrieve historical stock prices for the past year, and analyze the stock's performance.
We send this prompt to the agent using the chat
method, which processes the request and generates the desired code.
When this code is run, you’ll see output from the LLM that looks something like this:

Remember that LLMs are probabilistic so your output will look different.
Your next steps
Now that you have set up the environment and initiated a query to generate Python code, try changing the prompt.
Experiment with changing the date range to explore different time periods. This will help you understand how the code adapts to different inputs and deepens your understanding of stock data analysis.
