Technical analysis with Python: 3 indicators you can learn in 2.5 minutes

Technical analysis with Python: 3 indicators you can learn in 2.5 minutes
When I first started trading, I sat next to Eddie.
Eddie was on his second career. During his first, he had a few bad trades and blew up his trading account. He took some time off and started again.
Instead of relying on his instincts like the first time, he used technical analysis to get an edge.
I watched as he put levels into his spreadsheet, drew lines on the chart, and religiously bought at sold when the charts told him to.
Eddie always seemed to make money.
“Hey Eddie, what are those levels you always trade?” I asked one day.
He gave me his secret:
“Ah, it’s where all the traders think something’s going to happen.”
Then he walked away.
“Useless I thought.”
Only now do I realize what he was talking about.
Lines on charts are self-fulfilling prophecies and important to understand.
All traders see the same thing and often trade the same way.
This is what Eddie understood that most traders do not.
Today, you will use the popular TA-Lib technical analysis library to plot Bollinger Bands, RSI, and MACD using Python.
Traders use Bollinger Bands to identify potential trading opportunities in the financial markets.
They're usually used for identifying overbought and oversold conditions.
People also use them for measuring volatility and getting ahead of trend reversals.
Traders use the Relative Strength Index (RSI) to identify overbought or oversold conditions.
RSI oscillates between 0 and 100. Values above 70 show overbought conditions and values below 30 show oversold conditions.
The Moving Average Convergence Divergence (MACD) is a popular technical analysis tool, too. It's used by traders to identify trend reversals, momentum shifts, and trend strength.
MACD is based on the difference between two exponential moving averages with different periods.
Import and setup
Start by importing Matplotlib for plotting and pandas for data manipulation.
Then import TA-Lib and the OpenBB SDK.
1%matplotlib inline
2
3import matplotlib.pyplot as plt
4import pandas as pd
5from talib import RSI, BBANDS, MACD
6
7from openbb_terminal.sdk import openbb
8from openbb_terminal.sdk import TerminalStyle
9theme = TerminalStyle("light", "light", "light")
Use OpenBB to grab some stock data.
1data = (
2 openbb
3 .stocks
4 .load("AAPL", start_date="2020-01-01")
5 .rename(columns={"Adj Close": "close"})
6)
TA-Lib has more than 150 indicators and is one of the most popular libraries around. Using it is simple with Python.
1# Create Bollinger Bands
2up, mid, low = BBANDS(
3 data.close,
4 timeperiod=21,
5 nbdevup=2,
6 nbdevdn=2,
7 matype=0
8)
9
10# Create RSI
11rsi = RSI(data.close, timeperiod=14)
12
13# Create MACD
14macd, macdsignal, macdhist = MACD(
15 data.close,
16 fastperiod=12,
17 slowperiod=26,
18 signalperiod=9
19)
20
21Depending on the indicator, the function takes different values. BBANDS takes the closing price, the lookback window, and the number of standard deviations. RSI just takes the lookback window, and MACD takes the fast, slow, and signal periods.
Depending on the indicator, the function takes different values. BBANDS takes the closing price, the lookback window, and the number of standard deviations. RSI just takes the lookback window, and MACD takes the fast, slow, and signal periods.
Plot the indicators
Use pandas to put all the data together and Matplotlib to plot it. First the data.
1macd = pd.DataFrame(
2 {
3 "MACD": macd,
4 "MACD Signal": macdsignal,
5 "MACD History": macdhist,
6 }
7)
8
9data = pd.DataFrame(
10 {
11 "AAPL": data.close,
12 "BB Up": up,
13 "BB Mid": mid,
14 "BB down": low,
15 "RSI": rsi,
16 }
17)
Create two DataFrames: the first for the MACD signals, the second for the remaining indicators.
Then plot them.
1fig, axes = plt.subplots(
2 nrows=3,
3 figsize=(15, 10),
4 sharex=True
5)
6
7data.drop(["RSI"], axis=1).plot(
8 ax=axes[0],
9 lw=1,
10 title="BBANDS"
11)
12data["RSI"].plot(
13 ax=axes[1],
14 lw=1,
15 title="RSI"
16)
17axes[1].axhline(70, lw=1, ls="--", c="k")
18axes[1].axhline(30, lw=1, ls="--", c="k")
19macd.plot(
20 ax=axes[2],
21 lw=1,
22 title="MACD",
23 rot=0
24)
25axes[2].set_xlabel("")
26fig.tight_layout()
You created tree charts, all aligned by date. The top shows the Bollinger Bands, the middle one RSI, and the bottom the MACD.
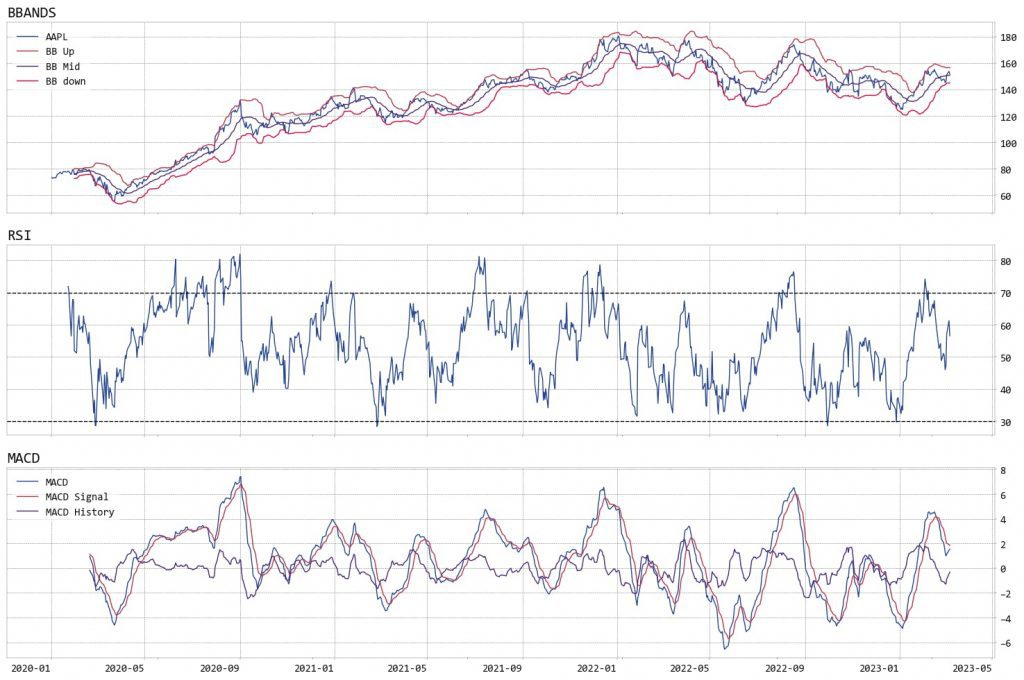
Since all traders see the same charts, you can use technical analysis to find out what other traders are doing.
Used with time series and other quantitative technicals, technical analysis can be used to filter out the noise from messy data.
