Web Development with Python: Flask and Django
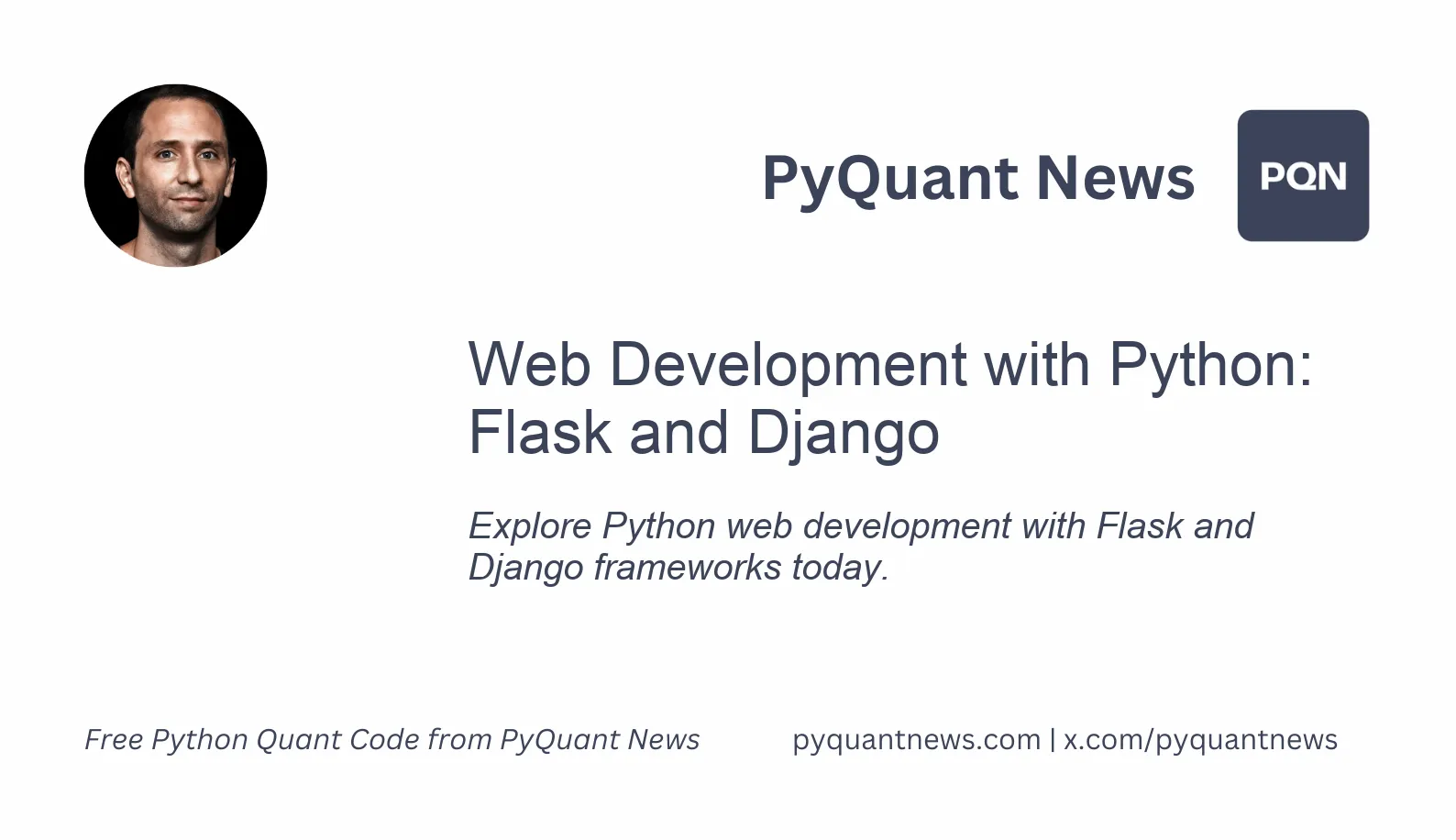
Web Development with Python: Flask and Django
In today's dynamic world of web development, Python has emerged as a preferred language for many developers. Its simplicity, readability, and extensive libraries make it a favored choice. Among the various Python web frameworks, Flask and Django stand out prominently. This guide delves into web development with Python, highlighting Flask and Django, and offers insights into building robust and scalable web applications.
The Rise of Python in Web Development
Python, created by Guido van Rossum in the late 1980s, has become extremely popular in fields like web development, data science, AI, and machine learning. Its clear syntax, dynamic typing, and extensive standard library appeal to developers. In the realm of web development, Python's versatility and strong community support have led to the creation of powerful frameworks such as Flask and Django.
Introduction to Flask
Flask, a micro-framework for Python developed by Armin Ronacher in 2010, is known for its lightweight and flexible nature. It's perfect for small to medium-sized applications. Flask's minimalist approach lets developers include only the components they need, streamlining the development process.
Key Features of Flask
- Simplicity and Flexibility: Flask's core philosophy promotes simplicity and flexibility. It provides essential tools for web development without imposing a rigid structure, giving developers full control over application design.
- Extensibility: Flask's modular design supports various plugins and extensions like Flask-SQLAlchemy for database integration and Flask-WTF for form handling.
- Built-in Development Server: Flask includes a development server for easy testing and debugging of applications.
- RESTful Request Dispatching: Flask supports RESTful request dispatching, making it suitable for APIs and efficient HTTP request handling.
Building a Simple Application with Flask
To illustrate Flask's basics, let's create a simple web application displaying a "Hello, World!" message.
Installation: Ensure Python is installed on your system. Then, install Flask using pip:
pip install Flask
Creating the Application: Create a file named app.py
and add the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
Running the Application: Execute the following command in your terminal:
python app.py
Open your browser and go to http://127.0.0.1:5000/
to see the "Hello, World!" message.
Introduction to Django
Django, a high-level Python web framework created by Adrian Holovaty and Simon Willison in 2005, promotes rapid development and clean design. It follows the "batteries-included" philosophy, offering a wide range of built-in features and tools, making it suitable for large-scale and complex applications.
Key Features of Django
- Robust Admin Interface: Django provides a customizable admin interface, making it easy to manage application data.
- ORM (Object-Relational Mapping): Django's ORM enables developers to interact with databases using Python code, simplifying SQL complexities.
- Security: Django includes built-in security features to protect against common vulnerabilities like SQL injection, XSS, and CSRF.
- Scalability: Designed to handle high-traffic applications, Django is ideal for large-scale projects.
- Template Engine: Django's template engine helps create dynamic HTML content by separating the presentation layer from the logic layer.
Building a Simple Application with Django
To understand Django better, let's create a simple web application displaying a list of books.
Installation: Ensure Python is installed, then install Django using pip:
pip install django
Creating a Project: Create a new Django project:
django-admin startproject booklist
Navigate to the project directory:
cd booklist
Creating an App: Create a new app within the project:
python manage.py startapp books
Defining Models: In books/models.py
, define a Book
model:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.CharField(max_length=100)
def __str__(self):
return self.title
Registering the App: Add the books
app to the INSTALLED_APPS
list in booklist/settings.py
:
INSTALLED_APPS = [
...
'books',
]
Running Migrations: Create and apply the database migrations:
python manage.py makemigrations
python manage.py migrate
Creating Views and Templates: Define a view to display the list of books in books/views.py
:
from django.shortcuts import render
from .models import Book
def book_list(request):
books = Book.objects.all()
return render(request, 'books/book_list.html', {'books': books})
Create a template to display the books in books/templates/books/book_list.html
:
<!DOCTYPE html>
<html>
<head>
<title>Book List</title>
</head>
<body>
<h1>Book List</h1>
<ul>
{% for book in books %}
<li>{{ book.title }} by {{ book.author }}</li>
{% endfor %}
</ul>
</body>
</html>
Configuring URLs: Add a URL pattern to route requests to the view in books/urls.py
:
from django.urls import path
from .views import book_list
urlpatterns = [
path('', book_list, name='book_list'),
]
Include the app's URLs in the project's urls.py
:
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('admin/', admin.site.urls),
path('books/', include('books.urls')),
]
Running the Development Server: Run the development server:
python manage.py runserver
Open your browser and go to http://127.0.0.1:8000/books/
to see the list of books.
Flask vs. Django: A Comparative Analysis
Both Flask and Django are powerful Python web frameworks, but they cater to different needs and preferences. Here is a comparative analysis to help you decide which framework suits your project best:
Flask
- Use Case: Ideal for small to medium-sized applications, APIs, and microservices.
- Flexibility: Offers greater flexibility and control over application design.
- Learning Curve: Easier for beginners due to its simplicity and minimalistic approach.
- Extensibility: Highly extensible with various plugins and extensions.
- Development Speed: Faster for projects that require only essential features.
Django
- Use Case: Suitable for large-scale applications and projects requiring a robust and scalable architecture.
- Batteries-Included: Comes with a wide range of built-in features and tools, reducing the need for third-party libraries.
- Learning Curve: Steeper learning curve due to its comprehensive features and conventions.
- Security: Includes built-in security features to protect against common web vulnerabilities.
- Admin Interface: Provides a powerful and customizable admin interface out-of-the-box.
Both Flask and Django have their strengths and are suited for different types of projects. Flask's flexibility and simplicity make it ideal for smaller applications and APIs, while Django's comprehensive feature set and scalability make it perfect for larger, more complex projects. Choose the framework that best aligns with your project requirements and development style.
Resources for Learning Flask and Django
For those looking to deepen their knowledge of Flask and Django, here are some valuable resources to get you started:
- Official Documentation:
- Flask: Flask Documentation
- Django: Django Documentation
- Books:
- Flask Web Development by Miguel Grinberg: A comprehensive guide to building web applications with Flask.
- Django for Beginners by William S. Vincent: An introductory book covering the basics of Django.
- Online Courses:
- Udemy: Offers in-depth tutorials and hands-on projects for both Flask and Django.
- Coursera: Courses on web development with Python, including Flask and Django, taught by experienced instructors.
- YouTube Channels:
- Corey Schafer: Excellent tutorials on both Flask and Django, covering a wide range of topics and practical examples.
- Community Forums:
- Stack Overflow: A valuable resource for getting answers to specific questions and troubleshooting issues related to Flask and Django development.
- Reddit: Subreddits such as r/flask and r/django provide a platform for developers to share knowledge and experiences.
These resources offer a mix of documentation, books, courses, and community support to help you master Flask and Django and apply them effectively in your projects.
Conclusion
Flask and Django are two of the most powerful and versatile frameworks for web development with Python. Understanding the basics of these frameworks and leveraging available resources can help developers create high-quality web applications that meet their specific needs. Whether you are a beginner or an experienced developer, exploring Flask and Django will undoubtedly enhance your web development skills and open new possibilities in web applications. Start your journey today and see where these powerful frameworks can take you.