Reinforcement Learning for Adaptive Trading Algorithms
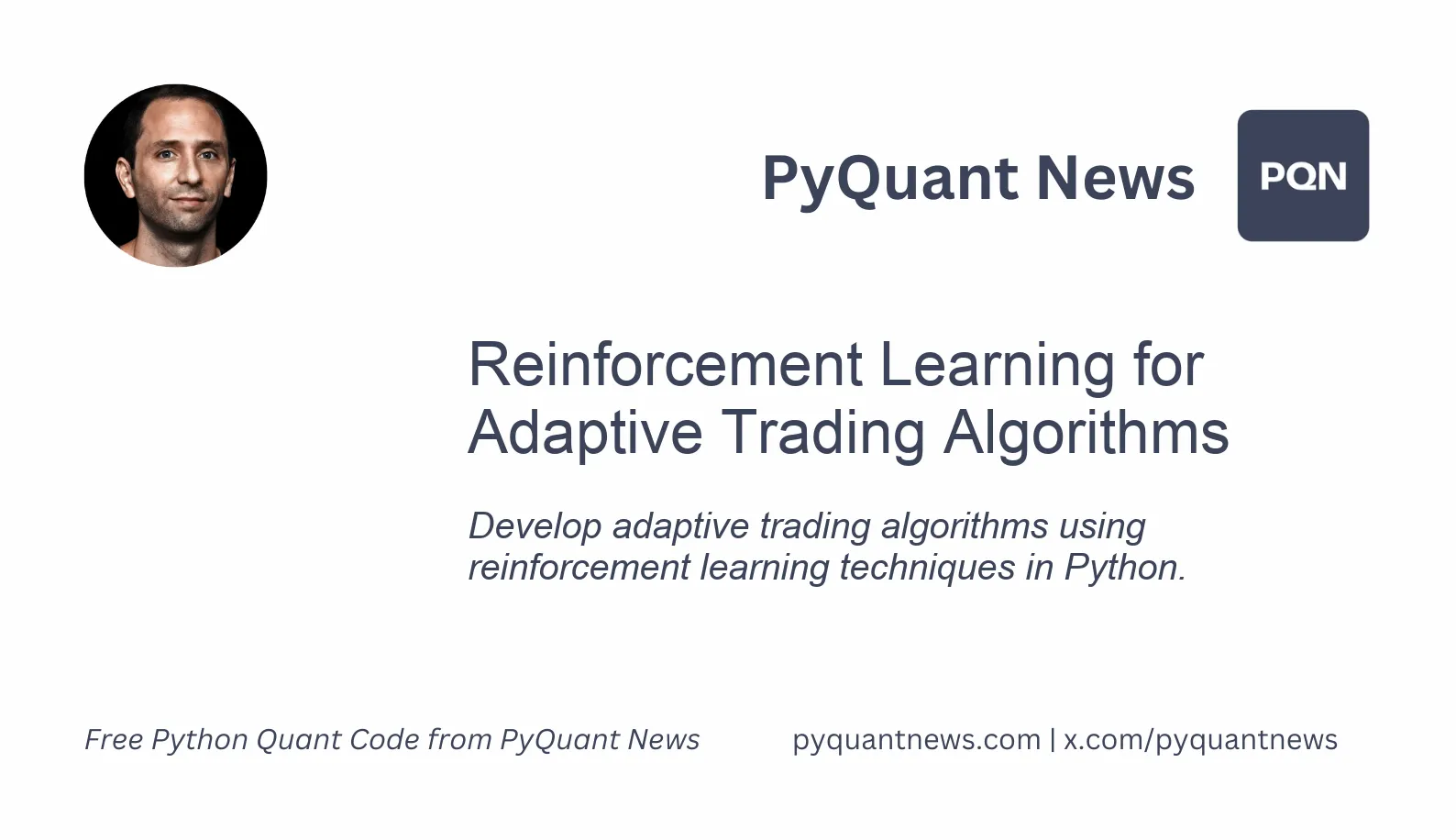
Reinforcement Learning for Adaptive Trading Algorithms
In today's fast-paced financial markets, developing adaptive trading algorithms is more important than ever. Financial institutions and independent traders are increasingly turning to reinforcement learning techniques in Python for their unmatched ability to adapt to dynamic market conditions and improve trading performance over time. This article explores how reinforcement learning techniques can be leveraged in Python to create sophisticated trading algorithms that evolve in real-time.
Understanding Reinforcement Learning
Reinforcement learning is a branch of machine learning where an agent learns to make decisions by performing actions in an environment to maximize cumulative reward. Unlike supervised learning, which relies on labeled datasets, reinforcement learning involves an agent learning through direct interaction with its environment. This makes it particularly suited for complex and dynamic tasks like trading.
Key Concepts
The key components of a reinforcement learning system include:
- Agent: The decision-maker.
- Environment: The domain in which the agent operates.
- Actions: The set of all possible moves the agent can make.
- States: The different configurations the environment can be in.
- Reward: The feedback received by the agent after performing an action.
Reinforcement Learning in Trading
In trading, the agent represents the trading algorithm, the environment is the financial market, actions include buy, sell, or hold decisions, states reflect various market conditions, and rewards are the resulting profits or losses.
Reinforcement learning is particularly advantageous for trading due to its ability to adapt to the unpredictable and fluctuating nature of financial markets. Traditional trading algorithms are often rule-based and static, limiting their effectiveness in changing market conditions. In contrast, reinforcement learning in trading enables algorithms to adapt and improve over time, potentially leading to better long-term performance.
Setting Up the Environment in Python
Python is a popular language for implementing reinforcement learning due to its extensive libraries and ease of use. Key libraries include TensorFlow, Keras, PyTorch, and OpenAI's Gym.
Install Necessary Libraries and Tools
Install the required libraries using pip:
pip install tensorflow keras gym numpy pandas
Define the Trading Environment
You can create a custom trading environment by extending the gym.Env
class. This involves defining the __init__
, step
, reset
, and render
methods.
import gym
from gym import spaces
import numpy as np
class TradingEnv(gym.Env):
def __init__(self, data):
super(TradingEnv, self).__init__()
self.data = data
self.action_space = spaces.Discrete(3) # Buy, Sell, Hold
self.observation_space = spaces.Box(low=-np.inf, high=np.inf, shape=(data.shape[1],), dtype=np.float32)
self.current_step = 0
def step(self, action):
self.current_step += 1
observation = self.data[self.current_step]
reward = self.calculate_reward(action)
done = self.current_step >= len(self.data) - 1
return observation, reward, done, {}
def reset(self):
self.current_step = 0
return self.data[self.current_step]
def render(self, mode='human'):
pass
def calculate_reward(self, action):
return 0 # Placeholder
Load Market Data
Use libraries like pandas
to load and preprocess historical market data. This data will be used to simulate the market environment.
import pandas as pd
data = pd.read_csv('market_data.csv')
Developing the Trading Algorithm
With the environment set up, the next step is to develop the trading algorithm. This involves choosing an RL algorithm, defining the neural network architecture, and training the agent.
Choosing an RL Algorithm
Selecting the right reinforcement learning algorithm is crucial. Popular choices include Q-learning, Deep Q-Networks (DQN), Policy Gradients, and Proximal Policy Optimization (PPO). Each has distinct advantages and is suited to different types of trading strategies.
Implementing the Neural Network
A neural network is used to approximate the Q-value function or the policy in RL. Below is an example of a simple neural network implemented using Keras.
from keras.models import Sequential
from keras.layers import Dense
from keras.optimizers import Adam
def build_model(state_size, action_size):
model = Sequential()
model.add(Dense(24, input_dim=state_size, activation='relu'))
model.add(Dense(24, activation='relu'))
model.add(Dense(action_size, activation='linear'))
model.compile(loss='mse', optimizer=Adam(lr=0.001))
return model
Training the Agent
Training involves interacting with the environment, collecting experiences, and using these experiences to update the model. The ReplayBuffer
is commonly used to store past experiences and sample mini-batches for training, ensuring that the learning process is stable and less correlated.
import random
class ReplayBuffer:
def __init__(self, max_size):
self.buffer = []
self.max_size = max_size
def add(self, experience):
if len(self.buffer) >= self.max_size:
self.buffer.pop(0)
self.buffer.append(experience)
def sample(self, batch_size):
return random.sample(self.buffer, batch_size)
Evaluating and Fine-Tuning the Algorithm
After training, it is essential to evaluate the agent's performance using comprehensive metrics like cumulative return, Sharpe ratio, and maximum drawdown to ensure it meets the desired trading objectives. Fine-tuning involves adjusting hyperparameters, refining the neural network architecture, and incorporating additional market features.
Challenges and Considerations
Despite its potential, developing RL-based trading algorithms presents several challenges:
- Data Quality: High-quality historical market data is essential for accurate model predictions and reliable performance.
- Computational Resources: Training deep learning models requires significant computational power.
- Overfitting: A model that performs well on past data may fail when exposed to new, unseen market conditions.
- Risk Management: Incorporating risk management techniques is crucial to prevent significant losses.
Resources for Further Learning
For those interested in diving deeper into reinforcement learning and its applications in trading, here are some valuable resources:
Books
- Reinforcement Learning: An Introduction by Richard S. Sutton and Andrew G. Barto
- Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow by Aurélien Géron
Online Courses
- Deep Reinforcement Learning Nanodegree by Udacity
- Coursera's Reinforcement Learning Specialization
Research Papers
- Playing Atari with Deep Reinforcement Learning by Mnih et al.
- Asynchronous Methods for Deep Reinforcement Learning by Mnih et al.
Github Repositories
- OpenAI Baselines: Provides high-quality implementations of RL algorithms.
- TensorTrade: A framework for developing and testing trading algorithms using RL.
Websites and Blogs
- Towards Data Science: Features articles on machine learning and reinforcement learning.
- QuantStart: Offers tutorials and articles on algorithmic trading.
Conclusion
Applying reinforcement learning techniques in Python for adaptive trading algorithms represents a significant leap forward in algorithmic trading. By utilizing reinforcement learning, traders can develop systems that not only execute trades but learn and adapt to ever-changing market conditions, potentially improving performance and profitability. While challenges remain, ongoing research and advancements in reinforcement learning hold great promise for the future, potentially leading to more robust and intelligent trading systems.