Quantitative Finance Techniques in Python
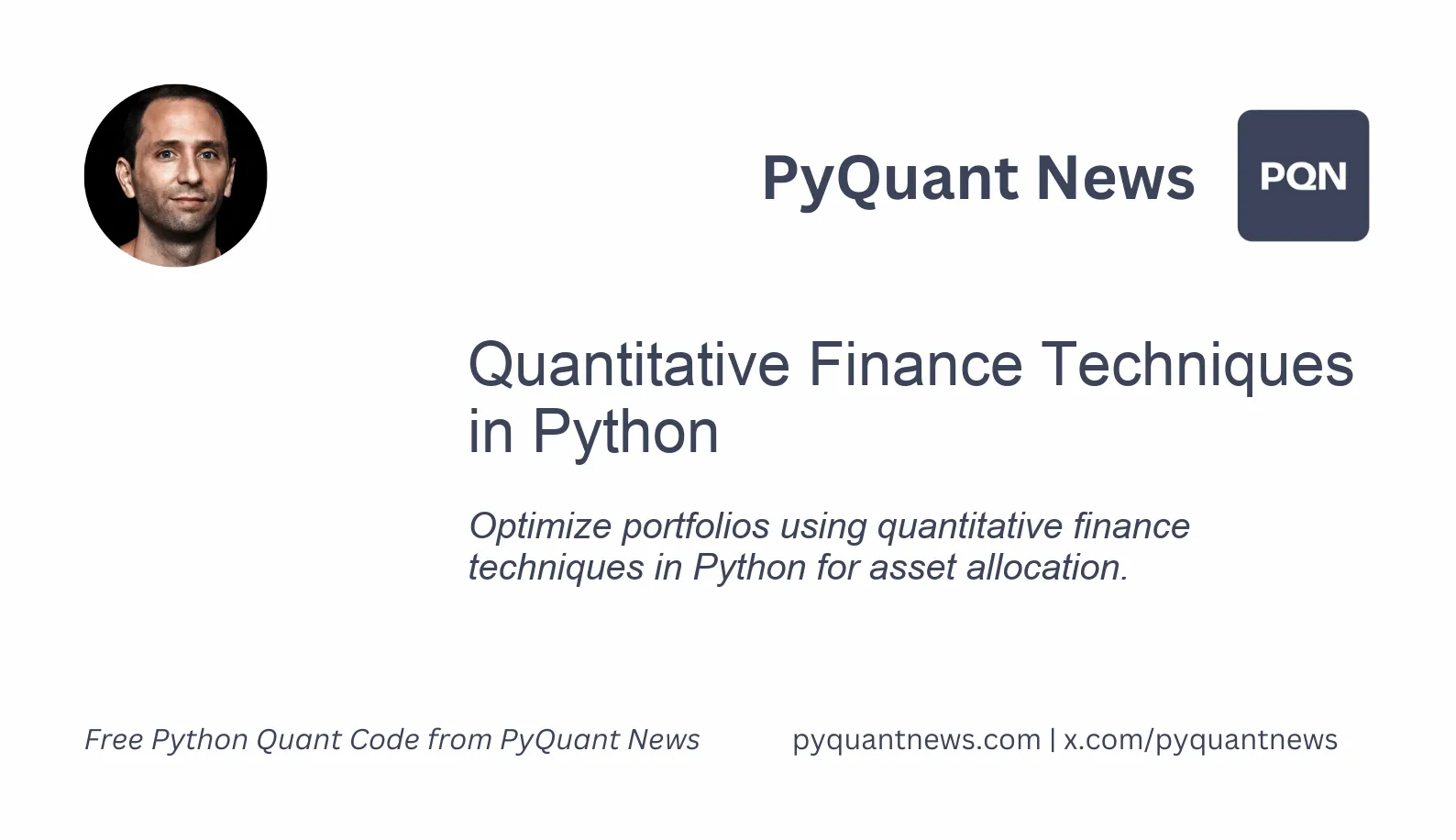
Quantitative Finance Techniques in Python
In today's fast-paced financial world, optimizing portfolios and allocating assets effectively is vital. Quantitative finance, leveraging mathematical models and computational tools, aims to achieve this. Python, with its powerful libraries and user-friendly nature, has become a top choice for financial analysts and data scientists. This article delves into using Python for portfolio optimization and asset allocation, highlighting key concepts and practical implementations.
By reading this article, you'll gain insights into Modern Portfolio Theory (MPT), the Capital Asset Pricing Model (CAPM), and the Efficient Frontier. You'll also learn how to use Python for portfolio optimization, making it a valuable resource for financial analysts, data scientists, and students seeking to enhance their investment strategies.
The Foundations of Quantitative Finance
Modern Portfolio Theory (MPT)
Modern Portfolio Theory, developed by Harry Markowitz in the 1950s, guides constructing a portfolio that maximizes expected return for a given level of risk. MPT emphasizes diversification to minimize risk.
Example: Consider two stocks, Stock A and Stock B. If Stock A rises when Stock B falls, combining them can reduce overall risk.
Assumptions:
- Investors are rational and risk-averse.
- Markets are efficient.
Capital Asset Pricing Model (CAPM)
CAPM describes the relationship between systematic risk and expected return for assets. It aids in pricing risky securities and estimating expected returns based on market risk.
Formula: [ \text{Expected Return} = R_f + \beta (R_m - R_f) ] Where:
- ( R_f ) = Risk-free rate
- ( \beta ) = Beta (measure of sensitivity to market movements)
- ( R_m ) = Market return
Limitations:
- Relies on a single risk factor.
- Assumes perfectly efficient markets.
Efficient Frontier
The efficient frontier depicts portfolios offering the highest expected return for a specified level of risk or the lowest risk for a given level of return.
Visual Representation:
Python Libraries for Quantitative Finance
Python's versatility and robust ecosystem make it ideal for quantitative finance. Key libraries include:
- NumPy: Numerical operations.
- Pandas: Data manipulation and analysis.
- Matplotlib/Seaborn: Data visualization.
- SciPy: Scientific computations.
- cvxopt: Convex optimization.
- PyPortfolioOpt: Portfolio optimization.
Data Acquisition and Preprocessing
Acquiring and preprocessing data is the first step in any quantitative finance project. Financial data can be sourced from platforms like Yahoo Finance using the yfinance
library.
import yfinance as yf
import pandas as pd
# Define stock tickers
tickers = ['AAPL', 'MSFT', 'GOOGL', 'AMZN', 'TSLA']
# Download historical data
data = yf.download(tickers, start="2020-01-01", end="2023-01-01")['Adj Close']
# Calculate daily returns
returns = data.pct_change().dropna()
Note: Calculating daily returns is essential for understanding stock performance and is crucial for portfolio optimization.
Portfolio Optimization with PyPortfolioOpt
PyPortfolioOpt simplifies portfolio optimization, supporting mean-variance optimization, the Black-Litterman model, and efficient frontier plotting.
Mean-Variance Optimization
Mean-variance optimization constructs a portfolio that maximizes return for a given risk level.
from pypfopt import EfficientFrontier, risk_models, expected_returns
# Calculate expected returns and sample covariance
mu = expected_returns.mean_historical_return(data)
S = risk_models.sample_cov(data)
# Optimize for maximum Sharpe ratio
ef = EfficientFrontier(mu, S)
weights = ef.max_sharpe()
cleaned_weights = ef.clean_weights()
print(cleaned_weights)
# Performance of the optimized portfolio
performance = ef.portfolio_performance(verbose=True)
Sharpe Ratio: A measure of risk-adjusted return.
Plotting the Efficient Frontier
Visualizing the efficient frontier helps understand the trade-off between risk and return.
from pypfopt import plotting
# Plot the efficient frontier
plotting.plot_efficient_frontier(ef, show_assets=True)
Advanced Techniques: Black-Litterman Model
The Black-Litterman model merges investor views with market equilibrium to generate expected returns, addressing some CAPM and mean-variance optimization limitations.
Implementation in Python
from pypfopt import BlackLittermanModel, risk_models
# Market cap of each asset
market_caps = {
'AAPL': 2.3e12,
'MSFT': 1.9e12,
'GOOGL': 1.5e12,
'AMZN': 1.6e12,
'TSLA': 800e9
}
# Market prior
delta = 0.025 # Risk aversion coefficient
S = risk_models.sample_cov(data)
bl = BlackLittermanModel(S, pi='market', market_caps=market_caps, delta=delta)
# Investor views
views = pd.DataFrame({
'AAPL': [0.02],
'MSFT': [0.015]
}, index=['view1'])
# Uncertainty (covariance matrix) of the views
omega = pd.DataFrame({
'view1': [0.0001]
}, index=['view1'])
# Update returns based on views
bl.update_view(views, omega)
# New posterior expected returns
mu_bl = bl.bl_returns()
Intuition: The Black-Litterman model incorporates personal views into market equilibrium returns, enhancing traditional mean-variance optimization.
Asset Allocation Strategies
After constructing an optimized portfolio, the next step is asset allocation. Different strategies can be used based on goals and risk tolerance.
Equal Weighting
Equal weighting assigns the same weight to each asset in the portfolio.
equal_weights = {ticker: 1/len(tickers) for ticker in tickers}
print(equal_weights)
Pros: Simple and straightforward.
Cons: Ignores individual asset risk and return characteristics.
Risk Parity
Risk parity allocates assets so each contributes equally to the portfolio's overall risk.
from pypfopt import objective_functions
# Optimize for risk parity
ef = EfficientFrontier(mu, S)
ef.add_objective(objective_functions.L2_reg, gamma=0.1)
weights = ef.efficient_risk(target_volatility=0.15)
cleaned_weights = ef.clean_weights()
print(cleaned_weights)
# Performance of the risk parity portfolio
performance = ef.portfolio_performance(verbose=True)
Pros: Balances risk contributions from different assets.
Cons: May lead to less diversified portfolios.
Minimum Variance
The minimum variance portfolio aims to minimize the portfolio's overall risk.
# Optimize for minimum variance
ef = EfficientFrontier(mu, S)
weights = ef.min_volatility()
cleaned_weights = ef.clean_weights()
print(cleaned_weights)
# Performance of the minimum variance portfolio
performance = ef.portfolio_performance(verbose=True)
Pros: Focuses on risk reduction.
Cons: May result in lower returns.
Challenges and Considerations
While quantitative finance techniques provide powerful tools for portfolio optimization, they come with challenges:
Data Quality
High-quality, accurate data is vital for reliable results.
Example: Inaccurate data can lead to incorrect return and risk calculations.
Model Risk
Models are simplifications of reality and may not capture all market dynamics.
Example: A model may perform well historically but fail in new market conditions.
Overfitting
Over-relying on historical data can lead to overfitting, where the model performs well on past data but poorly on new data.
Strategy: Use cross-validation and regularization techniques to reduce overfitting.
Market Assumptions
Many models assume market efficiency, which may not always hold true.
Example: During market crises, the assumption of normally distributed returns may fail.
Resources for Further Learning
For those eager to delve deeper into quantitative finance and Python, the following resources are invaluable:
- "Python for Finance" by Yves Hilpisch: A comprehensive introduction to financial modeling using Python, covering basic financial concepts to advanced quantitative techniques.
- Coursera: Machine Learning for Trading by Georgia Tech: A deep dive into using machine learning techniques for trading and portfolio management, with practical Python implementations.
- QuantInsti's EPAT (Executive Programme in Algorithmic Trading): A rigorous program covering various aspects of algorithmic trading, including quantitative finance, financial computing, and systematic trading strategies.
- QuantStart: An online resource with numerous articles, tutorials, and books on quantitative finance, algorithmic trading, and Python programming.
- PyPortfolioOpt Documentation: The official documentation for the PyPortfolioOpt library, providing detailed guides and examples for portfolio optimization.
Conclusion
Integrating quantitative finance techniques with Python offers a robust framework for portfolio optimization and asset allocation. By leveraging libraries like PyPortfolioOpt, financial analysts and data scientists can build efficient portfolios tailored to specific risk-return profiles. However, it's important to stay aware of the underlying assumptions and potential pitfalls of these models. With the right tools and knowledge, investors can make informed decisions to achieve their investment goals.
Experiment with the techniques discussed and start implementing them in your projects. The journey of mastering quantitative finance and Python is both challenging and rewarding. Happy investing!