Python Trading Simulators to Test Strategies
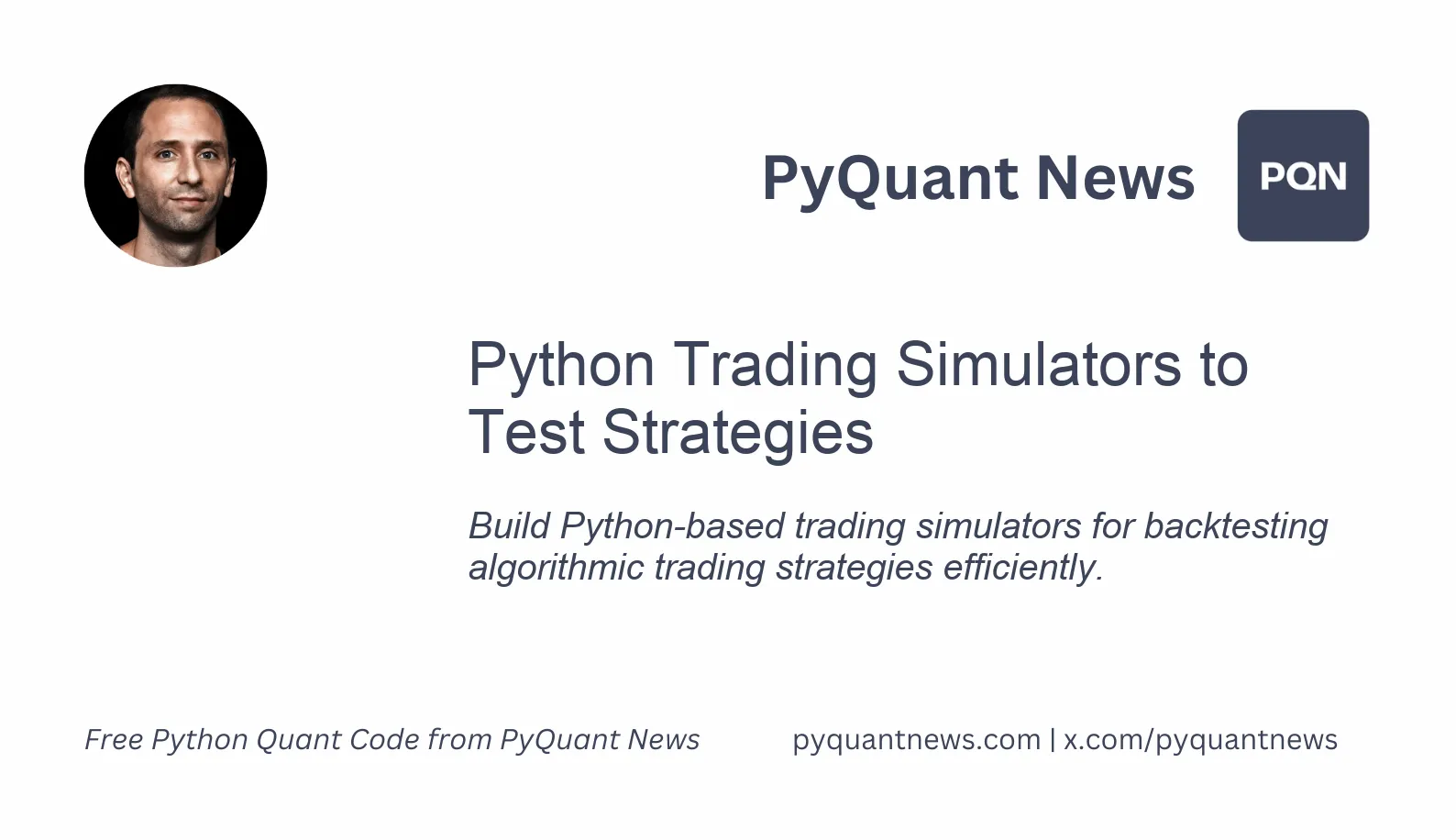
Python Trading Simulators to Test Strategies
In the high-stakes world of financial markets, a well-tested strategy can be the difference between profit and loss. With technology advancing rapidly, many traders are turning to algorithmic trading for a competitive edge. Before deploying any trading strategy in the real world, it's crucial to test its effectiveness in a controlled environment. This is where Python-based trading simulators come into play. These simulators allow traders to backtest trading strategies using historical data, ensuring they are robust and minimizing risks. This article delves into building Python-based trading simulators, focusing on tools, techniques, and best practices.
The Importance of Trading Simulators
Trading simulators act as a sandbox for traders to experiment with their strategies without risking real money. By simulating trades with historical data, traders can assess the performance of their strategies under various market conditions. This process, known as backtesting, helps identify potential flaws and allows for refinement before deploying strategies in live markets.
Benefits of Using Simulators:
- Risk-Free Environment: Test strategies without financial consequences.
- Performance Analysis: Gauge strategy effectiveness by analyzing historical data.
- Strategy Refinement: Tweak strategies and observe outcomes for continuous improvement.
- Market Condition Simulation: Test strategies under different conditions like bull and bear markets.
Getting Started with Python-Based Trading Simulators
Python is the go-to language for financial analysis and algorithmic trading due to its simplicity and extensive library support.
Step 1: Setting Up the Environment
Before coding, set up your development environment. Ensure Python is installed on your system. Download the latest version from the official Python website.
Next, install essential libraries using pip:
pip install pandas numpy matplotlib yfinance backtrader
- Pandas: For data manipulation and analysis.
- NumPy: For numerical computations.
- Matplotlib: For data visualization.
- yFinance: For fetching historical stock data.
- Backtrader: For backtesting trading strategies.
Step 2: Fetching Historical Data
To simulate trades, you'll need historical market data. The yFinance
library provides an easy way to fetch stock data from Yahoo Finance:
import yfinance as yf
# Fetch historical data for a specific stock (e.g., Apple)
data = yf.download('AAPL', start='2020-01-01', end='2021-01-01')
# Display the first few rows of the data
print(data.head())
Step 3: Building the Trading Simulator
With the data in hand, it's time to build the trading simulator using the Backtrader
library.
Defining the Strategy
Define your trading strategy. Here, we create a simple moving average crossover strategy:
import backtrader as bt
class SmaCross(bt.SignalStrategy):
def __init__(self):
sma1, sma2 = bt.ind.SMA(period=10), bt.ind.SMA(period=30)
crossover = bt.ind.CrossOver(sma1, sma2)
self.signal_add(bt.SIGNAL_LONG, crossover)
Setting Up the Cerebro Engine
Set up the Cerebro engine, the core of Backtrader:
cerebro = bt.Cerebro()
# Add the strategy
cerebro.addstrategy(SmaCross)
# Add the data feed
data_feed = bt.feeds.PandasData(dataname=data)
cerebro.adddata(data_feed)
# Set the initial capital
cerebro.broker.setcash(10000)
# Run the backtest
cerebro.run()
# Plot the results
cerebro.plot()
Analyzing Results
After running the backtest, analyze the results to understand your trading strategy's performance. Backtrader provides built-in analyzers for metrics like Sharpe ratio and drawdown:
# Add analyzers
cerebro.addanalyzer(bt.analyzers.SharpeRatio, _name='sharpe')
cerebro.addanalyzer(bt.analyzers.DrawDown, _name='drawdown')
# Run the backtest
results = cerebro.run()
# Extract the analyzers
sharpe = results[0].analyzers.sharpe.get_analysis()
drawdown = results[0].analyzers.drawdown.get_analysis()
print(f'Sharpe Ratio: {sharpe["sharperatio"]}')
print(f'Max Drawdown: {drawdown["max"]["drawdown"]}%')
Understanding Performance Metrics
- Sharpe Ratio: Measures the risk-adjusted return of the strategy. A higher Sharpe ratio indicates better risk-adjusted performance.
- Drawdown: Represents the peak-to-trough decline in portfolio value. Understanding drawdown helps in assessing the strategy's risk.
Refining the Strategy
Based on the analysis, refine your strategy. Adjust parameters, add filters, or try different indicators to improve performance. Repeat the backtesting process to evaluate the changes.
Advanced Techniques
While the basic setup provides a solid foundation, advanced techniques can further enhance the simulator's capabilities.
Walk-Forward Analysis
Walk-forward analysis involves dividing the data into training and testing periods. Optimize the strategy on the training period and then test it on the testing period. Repeat this process multiple times to ensure robustness.
from sklearn.model_selection import TimeSeriesSplit
# Split the data into training and testing periods
tscv = TimeSeriesSplit(n_splits=5)
for train_index, test_index in tscv.split(data):
train_data = data.iloc[train_index]
test_data = data.iloc[test_index]
# Set up and run the backtest for each split
cerebro.adddata(bt.feeds.PandasData(dataname=train_data))
cerebro.run()
Monte Carlo Simulation
Monte Carlo simulation involves running the strategy multiple times with varying parameters to assess its performance under different scenarios. This technique helps identify the strategy's sensitivity to parameter changes.
import random
# Define parameter ranges
param_ranges = {
'short_period': range(5, 20),
'long_period': range(20, 50)
}
# Run multiple simulations
for _ in range(100):
short_period = random.choice(param_ranges['short_period'])
long_period = random.choice(param_ranges['long_period'])
# Define the strategy with selected parameters
class SmaCross(bt.SignalStrategy):
def __init__(self):
sma1, sma2 = bt.ind.SMA(period=short_period), bt.ind.SMA(period=long_period)
crossover = bt.ind.CrossOver(sma1, sma2)
self.signal_add(bt.SIGNAL_LONG, crossover)
# Run the backtest
# ...
Resources for Further Learning
- Backtrader Documentation: Comprehensive guides and examples for building and backtesting trading strategies.
- Python for Finance by Yves Hilpisch: Covers the use of Python for financial analysis and algorithmic trading, including backtesting strategies.
- QuantConnect: A cloud-based platform for backtesting and deploying algorithmic trading strategies. Supports multiple languages, including Python.
- QuantStart: Offers tutorials and courses on quantitative trading and strategy development using Python.
- Algorithmic Trading and Finance Models with Python, R, and Stata by James Ma Weiming: A comprehensive guide to developing algorithmic trading strategies using Python, R, and Stata.
Conclusion
In summary, Python-based trading simulators offer a robust platform for backtesting and refining trading strategies. By leveraging libraries like Pandas, NumPy, Matplotlib, yFinance, and Backtrader, traders can perform thorough analyses and optimizations. Mastering these tools can significantly enhance your trading success. Ready to take your trading strategies to the next level? Start building your own Python-based trading simulator today and explore the endless possibilities of algorithmic trading.