Python Syntax, Keywords, and Constructs
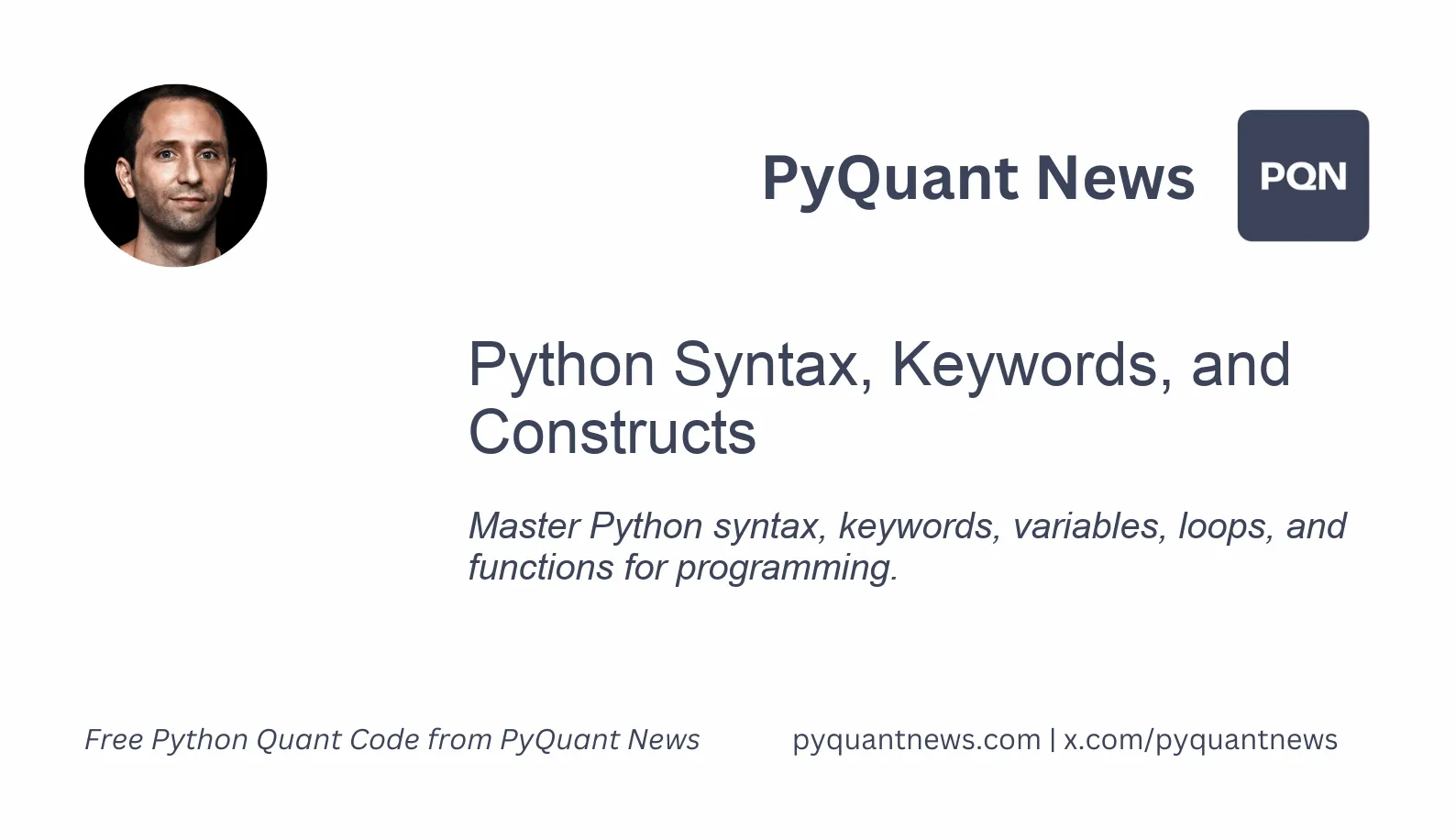
Python Syntax, Keywords, and Constructs
Python has earned a prestigious reputation in the vast world of programming languages due to its simplicity, readability, and versatility. Whether you're a beginner or an experienced developer, understanding Python syntax, keywords, and core constructs like loops and conditionals is vital. This article will explore these areas in depth, equipping you with the knowledge needed to harness Python's full power.
The Elegance of Python Syntax
Python syntax is designed to be clean and easy to read, making it an excellent choice for both beginners and experts. The language uses indentation to define code blocks instead of braces or keywords.
Indentation
Python uses indentation to delimit blocks of code. This approach reduces visual clutter, enhances readability, and enforces a uniform coding style.
if True:
print("Hello, World!")
In this example, the indented print
statement is part of the if
block. Consistent indentation ensures that code remains readable and logically structured.
Comments
Comments are crucial for writing understandable code. Python supports single-line comments using the #
symbol.
# This is a single-line comment
print("Hello, World!") # This prints a message
For multi-line comments, Python conventionally uses triple quotes ("""
or '''
).
"""
This is a multi-line comment.
It can span multiple lines.
"""
Use comments wisely to maintain code readability without over-cluttering.
Keywords: The Building Blocks
Keywords are reserved words in Python with special meanings that cannot be used as variable names. They form the foundation of Python's syntax and control structures.
Common Keywords
and
,or
,not
: Logical operators.if
,elif
,else
: Conditional statements.for
,while
: Looping constructs.def
: Defines a function.class
: Defines a class.try
,except
,finally
: Exception handling.
Mastering these keywords is essential for effective Python programming. Here is a brief overview of their usage:
if condition:
# code to execute if condition is true
elif another_condition:
# code to execute if another_condition is true
else:
# code to execute if all previous conditions are false
Logical Operators
Logical operators perform logical operations on conditional statements:
a = True
b = False
if a and b:
print("Both are True")
elif a or b:
print("At least one is True")
else:
print("Both are False")
Basic Programming Constructs
Variables
Variables store data values. In Python, you don’t need to declare the type of a variable explicitly; it is inferred from the value assigned.
x = 5
y = "Hello"
z = 3.14
Python's dynamic typing allows variable types to change.
Data Types
Python supports several data types, including integers, floats, strings, and booleans. Lists, tuples, sets, and dictionaries are also fundamental data structures.
# List: Ordered, mutable sequence of elements
my_list = [1, 2, 3]
# Tuple: Ordered, immutable sequence of elements
my_tuple = (1, 2, 3)
# Dictionary: Unordered collection of key-value pairs
my_dict = {"key1": "value1", "key2": "value2"}
# Set: Unordered collection of unique elements
my_set = {1, 2, 3}
Conditionals
Conditionals allow you to execute code based on certain conditions using if
, elif
, and else
statements.
age = 18
if age < 18:
print("Minor")
elif age == 18:
print("Just turned adult")
else:
print("Adult")
Loops
Loops are pivotal for performing repetitive tasks. Python supports for
and while
loops.
For Loop
The for
loop iterates over a sequence (such as a list, tuple, or string).
for i in range(5):
print(i)
While Loop
The while
loop continues executing as long as a condition remains true.
count = 0
while count < 5:
print(count)
count += 1
Functions
Functions are reusable pieces of code that perform a specific task. They are defined using the def
keyword.
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Exception Handling
Python provides robust mechanisms for handling exceptions using try
, except
, and finally
blocks.
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
finally:
print("Execution completed")
Real-World Applications
Understanding Python syntax, keywords, and constructs is not just academic. These elements are the bedrock of writing efficient, readable, and maintainable code in real-world applications. From web development using frameworks like Django and Flask to data analysis with libraries like Pandas and NumPy, Python’s versatility is unmatched.
Web Development
Python is a popular choice for web development, thanks to frameworks like Django and Flask. For instance, Django emphasizes rapid development and clean, pragmatic design.
# views.py in Django
from django.shortcuts import render
def home(request):
return render(request, 'home.html')
Data Analysis
Python’s rich ecosystem of libraries makes it a powerful tool for data analysis. Libraries like Pandas and NumPy offer functionalities to manipulate and analyze large datasets effortlessly.
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charles'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
Learning Resources
For those inclined to learn more about Python syntax, keywords, and programming constructs, several high-quality resources can aid in your journey:
Official Python Documentation
Official Python Documentation provides a comprehensive reference for all aspects of Python programming. It is an invaluable resource for both beginners and experienced developers.
Automate the Boring Stuff with Python
Automate the Boring Stuff with Python by Al Sweigart focuses on practical applications of Python, making it ideal for beginners looking to automate everyday tasks.
Python Crash Course
Python Crash Course by Eric Matthes offers a fast-paced introduction to Python. It covers basic concepts and extends to more advanced topics like web development and data visualization.
Codecademy’s Python Course
Codecademy’s Python Course provides an interactive learning experience suitable for beginners. It includes quizzes and projects to reinforce learning.
Real Python
Real Python is a treasure trove of tutorials, articles, and resources. It covers a wide array of topics, from basic syntax to advanced concepts like machine learning and web development.
Conclusion
Python’s simplicity and readability, coupled with its powerful constructs, make it an ideal language for both beginners and seasoned developers. Understanding its syntax, keywords, and basic programming constructs is the first step towards unleashing Python's full potential. The resources mentioned above will serve as valuable guides, helping you master the language and apply it to solve real-world problems.
Start your journey with Python, and you'll soon discover its elegance and power, enabling you to develop solutions that are both sophisticated and efficient. Happy coding!