Python in High-Frequency Trading: Low-Latency Techniques
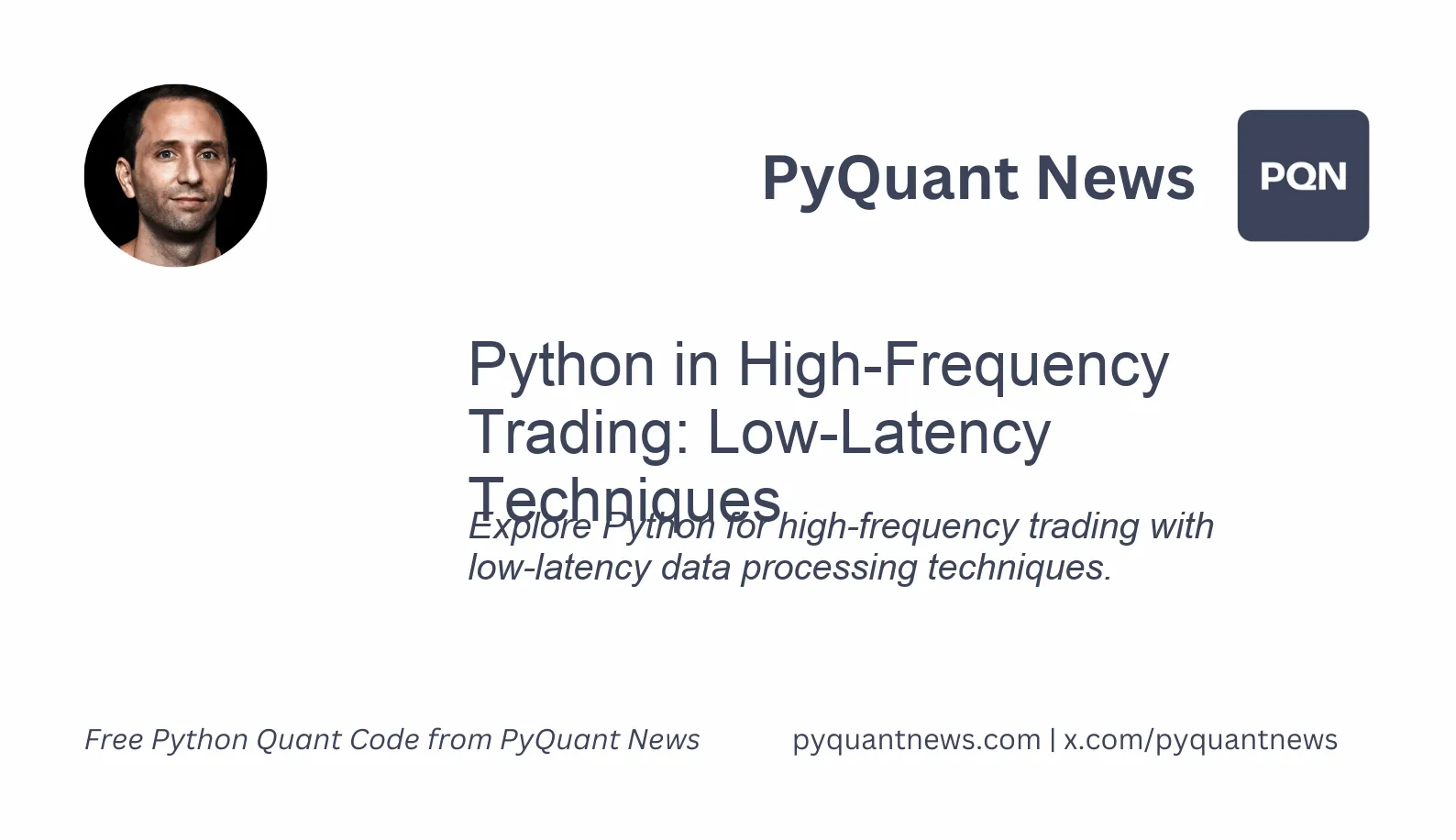
Python in High-Frequency Trading: Low-Latency Techniques
In the fast-paced world of financial markets, milliseconds can translate to millions. High-frequency trading (HFT) utilizes advanced algorithmic trading strategies to execute vast volumes of trades at remarkable speeds. Central to the success of HFT systems is their ability to process large data volumes with minimal latency. Python, celebrated for its extensive libraries and ease of use, has emerged as a favored language for developing these sophisticated trading systems. This article delves into using Python for HFT, emphasizing low-latency data processing techniques that make such systems effective.
The Rise of High-Frequency Trading
HFT involves using sophisticated algorithms to trade financial securities at extremely high speeds. HFT firms leverage cutting-edge technologies to analyze market data and execute trades within microseconds. This rapid trading strategy allows firms to capitalize on minute price discrepancies, achieving profits that accumulate over numerous trades.
Why Python?
Python has gained significant traction in the financial industry due to its simplicity, readability, and extensive ecosystem of libraries. While traditionally, languages like C++ have been preferred for HFT due to their performance advantages, Python's versatility and rapid development cycle have made it a formidable contender in the realm of high-frequency trading.
Low-Latency Data Processing: The Heart of HFT
At the core of HFT systems lies the ability to process data with minimal latency. Low-latency data processing ensures that trading algorithms receive and act on market data faster than competitors. Here are key techniques and tools in Python that enable this:
Efficient Data Structures and Algorithms
While Python's built-in data structures such as lists, dictionaries, and sets are optimized for performance, HFT systems often require more specialized structures. Libraries like NumPy and pandas provide efficient array and DataFrame structures that facilitate fast data manipulation and analysis.
- NumPy: NumPy is a fundamental library for numerical computing in Python. It provides support for arrays, matrices, and a suite of mathematical functions. NumPy arrays are more efficient than Python lists for large datasets, making them ideal for handling real-time market data.
- pandas: pandas is a powerful data manipulation library that provides data structures like Series and DataFrames. It is designed for handling time-series data, which is crucial for HFT. Using pandas, traders can quickly filter, aggregate, and transform market data.
Asynchronous Programming
In HFT, the ability to handle multiple tasks concurrently is important for maintaining low latency. Python's asynchronous programming capabilities, introduced in Python 3.4 with the asyncio
library, allow for non-blocking execution of tasks.
- asyncio: This library provides an event loop that enables asynchronous I/O operations, ensuring that the system can process new data while waiting for network responses or disk I/O. This reduces the time wasted on waiting and improves overall system performance.
Just-In-Time Compilation
Python's interpreted nature can introduce performance bottlenecks. Just-In-Time (JIT) compilation, offered by libraries like Numba and PyPy, can significantly enhance execution speed.
- Numba: Numba is a JIT compiler that translates Python code into machine code at runtime. By annotating functions with Numba's
@jit
decorator, critical sections of the code can be compiled for faster execution. - PyPy: PyPy is an alternative implementation of Python that includes a JIT compiler. It can execute Python code much faster than the standard CPython interpreter.
High-Performance Networking
In HFT, receiving market data and sending orders with minimal delay is paramount. Python provides several libraries for high-performance networking.
- ZeroMQ: ZeroMQ is a high-performance asynchronous messaging library that supports various communication patterns. It is widely used in HFT systems for inter-process communication and data distribution.
- Redis: Redis is an in-memory data structure store that can be used as a message broker. Its pub/sub functionality allows for fast and efficient data distribution, making it suitable for real-time trading applications.
Building a Python-Based HFT System
Constructing an HFT system involves several components, each requiring meticulous attention to ensure low latency and high performance. Here is a high-level overview of the key components and how Python can be used to implement them:
Market Data Ingestion
The first step in an HFT system is the ingestion of market data. This involves connecting to market data feeds, receiving updates, and storing them for analysis. Python's networking libraries, such as ZeroMQ and asyncio, can be used to establish connections to market data providers and handle incoming data streams.
import zmq
import asyncio
context = zmq.Context()
socket = context.socket(zmq.SUB)
socket.connect("tcp://market-data-provider:port")
socket.setsockopt_string(zmq.SUBSCRIBE, "")
async def ingest_market_data():
while True:
data = await socket.recv()
process_market_data(data)
asyncio.run(ingest_market_data())
Data Processing and Analysis
Once market data is ingested, it must be processed and analyzed in real-time. This involves filtering, aggregating, and transforming the data to extract meaningful insights. Libraries like NumPy and pandas are invaluable for this purpose.
import pandas as pd
def process_market_data(data):
df = pd.DataFrame(data)
# Perform data analysis and transformation
# ...
Trading Algorithms
The heart of an HFT system is the trading algorithm. This is where the magic happens, as sophisticated algorithms analyze market data and make trading decisions. Python's rich ecosystem of libraries, such as scikit-learn for machine learning and statsmodels for statistical analysis, can be leveraged to develop and backtest trading strategies.
from sklearn.ensemble import RandomForestClassifier
def train_trading_model(data):
X = data[['feature1', 'feature2']]
y = data['target']
model = RandomForestClassifier()
model.fit(X, y)
return model
def execute_trades(model, data):
predictions = model.predict(data)
# Execute trades based on predictions
# ...
Order Execution
Once trading decisions are made, orders must be sent to the market with minimal delay. Python's networking libraries, such as ZeroMQ and Redis, can be used to send orders to the exchange.
import redis
r = redis.Redis(host='order-execution-server', port=6379, db=0)
def send_order(order):
r.publish('orders', order)
Monitoring and Logging
Monitoring and logging are essential for ensuring the system's reliability and performance. Python's logging module and monitoring tools like Prometheus can be used to track system metrics and log important events.
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def log_event(event):
logger.info(event)
Challenges and Considerations
While Python offers numerous advantages for HFT development, several challenges and considerations must be kept in mind:
- Performance: Despite the availability of JIT compilers, Python may still lag behind lower-level languages like C++ in terms of raw performance. Critical sections of the code may need to be optimized or offloaded to compiled languages.
- Garbage Collection: Python's garbage collector can introduce latency spikes. Careful memory management and profiling are necessary to minimize its impact.
- Concurrency: Python's Global Interpreter Lock (GIL) can be a limitation for multi-threaded applications. Using multiprocessing or asynchronous programming can help mitigate this.
Resources for Further Learning
For those interested in diving deeper into the world of HFT and Python, here are some valuable resources:
- Books:
- "Python for Finance: Analyze Big Financial Data" by Yves Hilpisch: This book provides a comprehensive introduction to using Python for financial data analysis and algorithmic trading.
- "Algorithmic Trading: Winning Strategies and Their Rationale" by Ernest P. Chan: A practical guide to developing and implementing trading strategies, with a focus on Python.
- Online Courses:
- Coursera's "Financial Engineering and Risk Management" by Columbia University: This course covers the principles of financial engineering and risk management, with practical applications using Python.
- Udemy's "Algorithmic Trading with Python and QuantConnect" by Jose Portilla: A hands-on course that teaches algorithmic trading using Python and the QuantConnect platform.
- Websites and Blogs:
- QuantStart: A website dedicated to quantitative finance and algorithmic trading, offering articles, tutorials, and resources for Python-based trading.
- QuantInsti Blog: A blog that covers various aspects of algorithmic trading, including Python programming, trading strategies, and market analysis.
- Open Source Projects:
- Zipline: An open-source backtesting library for Python, used to simulate trading strategies and analyze their performance.
- PyAlgoTrade: A Python library for backtesting and trading, designed for algorithmic trading strategies.
Conclusion
Python's versatility, combined with its extensive ecosystem of libraries and tools, makes it a powerful choice for developing high-frequency trading systems. By leveraging efficient data structures, asynchronous programming, JIT compilation, and high-performance networking, Python can achieve the low-latency data processing required for HFT. While challenges remain, the growing community and wealth of resources available make Python an increasingly attractive option for traders and developers alike. As the financial markets continue to evolve, Python's role in shaping the future of high-frequency trading is poised to expand, offering new opportunities for innovation and profit.