Python for Cryptocurrency Trading Algorithms
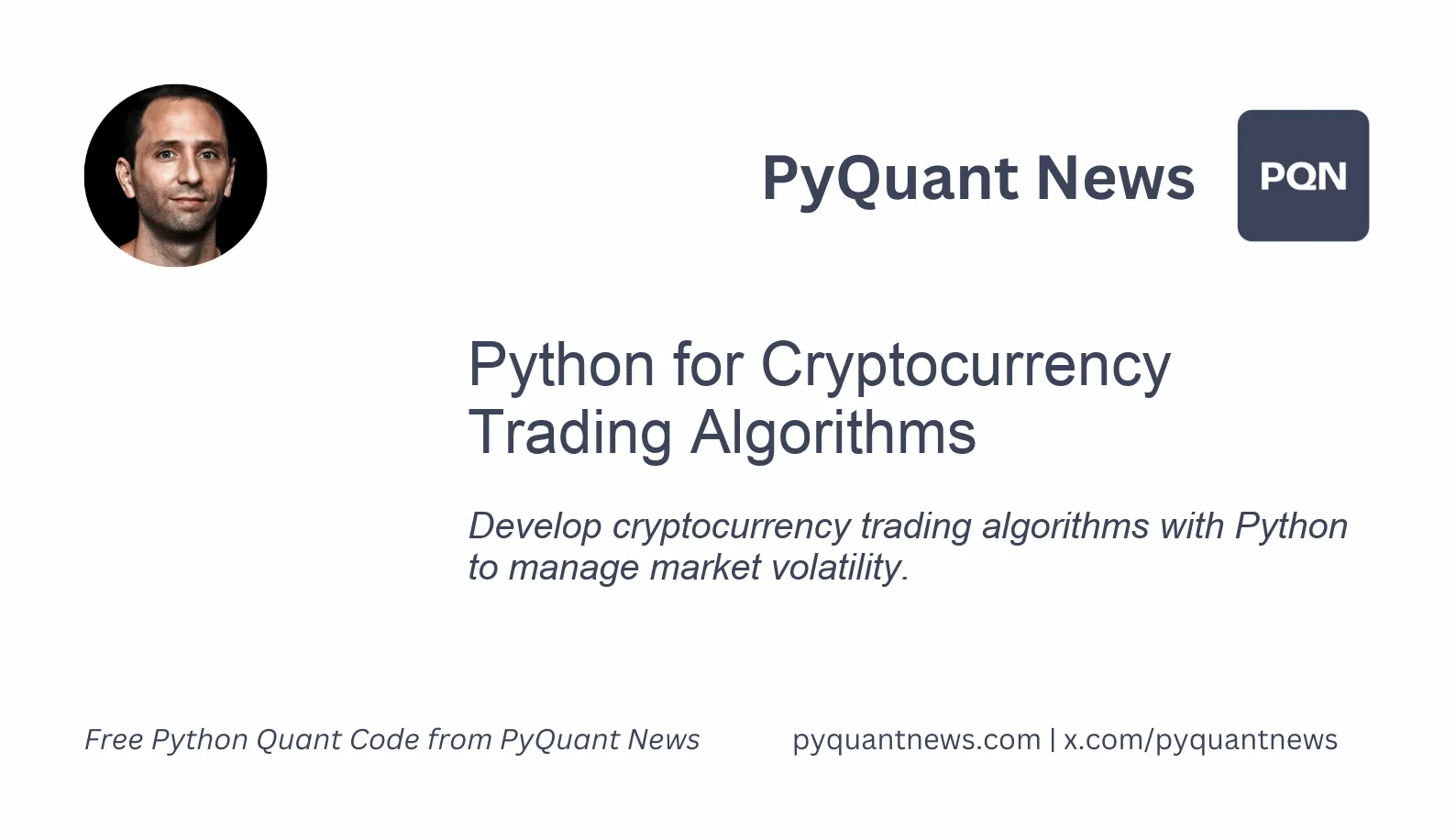
Python for Cryptocurrency Trading Algorithms
In the fast-paced cryptocurrency market, prices can fluctuate significantly within hours, creating both challenges and opportunities for traders. Python, an accessible and versatile programming language, has emerged as a powerful tool for developing cryptocurrency trading algorithms and strategies. Many successful cryptocurrency hedge funds leverage Python-based algorithms to exploit market inefficiencies. This article explores how to use Python for cryptocurrency trading, offering valuable insights for both novice and experienced traders.
The Allure of Cryptocurrency Trading
Cryptocurrencies like Bitcoin, Ethereum, and various altcoins have revolutionized financial markets. Unlike traditional markets, cryptocurrencies operate 24/7, presenting unique opportunities and challenges. The potential for significant profits attracts many traders, but the inherent volatility requires robust strategies to manage risks and capitalize on market movements.
Why Python?
Python has become the preferred language in the financial sector, including cryptocurrency trading, for several compelling reasons:
- Ease of Learning and Use: Python's clear and readable syntax makes it accessible for beginners and powerful for advanced users.
- Extensive Libraries: Python offers a vast array of libraries like NumPy, pandas, and Matplotlib for data analysis and visualization, and specialized libraries like TA-Lib for technical analysis.
- Community and Support: Python has a strong and active community, providing support, resources, and continuous updates.
- Integration Capabilities: Python easily integrates with other languages and tools, making it flexible for various trading platform requirements.
Steps to Develop Cryptocurrency Trading Algorithms with Python
Creating a robust trading algorithm involves a structured approach, incorporating data collection, analysis, strategy development, backtesting, and execution.
Data Collection
High-quality data is the foundation of any trading algorithm. In cryptocurrency trading, this involves collecting historical price data, trading volumes, and other relevant metrics from various exchanges. Python's libraries such as ccxt
are invaluable for this purpose.
import ccxt
import pandas as pd
exchange = ccxt.binance()
symbol = 'BTC/USDT'
timeframe = '1h'
since = exchange.parse8601('2020-01-01T00:00:00Z')
limit = 1000
all_data = []
while True:
data = exchange.fetch_ohlcv(symbol, timeframe, since, limit)
if not data:
break
since = data[-1][0] + 1
all_data += data
df = pd.DataFrame(all_data, columns=['timestamp', 'open', 'high', 'low', 'close', 'volume'])
df['timestamp'] = pd.to_datetime(df['timestamp'], unit='ms')
Data Analysis
With the data collected, the next step is to analyze it to identify patterns and trends. Python libraries such as pandas and NumPy are instrumental in this phase.
import numpy as np
# Calculating moving averages
df['SMA_50'] = df['close'].rolling(window=50).mean()
df['SMA_200'] = df['close'].rolling(window=200).mean()
# Identifying crossover points
df['Signal'] = np.where(df['SMA_50'] > df['SMA_200'], 1, 0)
df['Position'] = df['Signal'].diff()
Strategy Development
Strategy development involves creating rules for when to enter and exit trades. This can range from simple moving average crossovers to more complex machine learning-based models.
Example Strategy: Moving Average Crossover
A classic strategy uses moving averages to determine buy and sell signals. When a short-term moving average crosses above a long-term moving average, it's a buy signal. Conversely, when it crosses below, it's a sell signal.
def moving_average_crossover(df):
df['Short_MA'] = df['close'].rolling(window=50).mean()
df['Long_MA'] = df['close'].rolling(window=200).mean()
df['Signal'] = 0
df['Signal'][50:] = np.where(df['Short_MA'][50:] > df['Long_MA'][50:], 1, 0)
df['Position'] = df['Signal'].diff()
return df
df = moving_average_crossover(df)
Backtesting
Backtesting evaluates the effectiveness of a strategy by running it on historical data to see how it would have performed.
initial_balance = 10000
balance = initial_balance
positions = 0
for i in range(len(df)):
if df['Position'].iloc[i] == 1: # Buy Signal
positions = balance / df['close'].iloc[i]
balance = 0
elif df['Position'].iloc[i] == -1: # Sell Signal
balance = positions * df['close'].iloc[i]
positions = 0
final_balance = balance + positions * df['close'].iloc[-1]
print(f"Initial Balance: ${initial_balance}")
print(f"Final Balance: ${final_balance}")
Execution
Once a strategy has been backtested and optimized, the next step is executing it in the live market. This requires integrating with trading platforms via APIs.
import ccxt
exchange = ccxt.binance({
'apiKey': 'YOUR_API_KEY',
'secret': 'YOUR_API_SECRET',
})
def execute_trade(symbol, side, amount):
order = exchange.create_order(symbol, 'market', side, amount)
return order
# Example: Buying 0.01 BTC
execute_trade('BTC/USDT', 'buy', 0.01)
Managing Volatility
Cryptocurrency markets are highly volatile. Effective risk management strategies are essential to protect investments.
Diversification
Diversifying across different cryptocurrencies can help mitigate risks. While diversification doesn't eliminate risk, it can reduce the impact of a poor-performing asset.
Stop-Loss and Take-Profit Orders
Stop-loss and take-profit orders automate exits at predetermined price levels, protecting against significant losses and securing profits.
Position Sizing
Position sizing involves determining the amount of capital to allocate to each trade. A common approach is the 1% rule, where no more than 1% of the trading capital is risked on a single trade.
Advanced Strategies and Techniques
Machine Learning
Machine learning can be employed to develop more sophisticated trading algorithms. Libraries like scikit-learn and TensorFlow facilitate the development of predictive models.
from sklearn.ensemble import RandomForestClassifier
# Example: Predicting price direction
X = df[['SMA_50', 'SMA_200']].iloc[200:]
y = np.where(df['close'].iloc[200:].shift(-1) > df['close'].iloc[200:], 1, 0)
model = RandomForestClassifier()
model.fit(X, y)
df['Prediction'] = model.predict(X)
Sentiment Analysis
Sentiment analysis involves analyzing social media and news to gauge market sentiment. Libraries like TextBlob and NLTK can be used for this purpose.
from textblob import TextBlob
# Example: Sentiment analysis of tweets
tweets = ['Bitcoin is going to the moon!', 'Crypto market is crashing']
sentiments = [TextBlob(tweet).sentiment.polarity for tweet in tweets]
average_sentiment = sum(sentiments) / len(sentiments)
print(f"Average Sentiment: {average_sentiment}")
Resources for Further Learning
For those looking to deepen their understanding and skills in developing cryptocurrency trading algorithms with Python, here are some excellent resources:
- CCXT Library Documentation: Comprehensive documentation for the CCXT library, which provides access to numerous cryptocurrency exchanges.
- QuantStart: Offers a wealth of articles and tutorials on algorithmic trading, including Python-specific content.
- Python for Finance by Yves Hilpisch: A highly-regarded book that covers various aspects of financial analysis and algorithmic trading using Python.
- Algorithmic Trading and Quantitative Analysis using Python: A Coursera course that provides structured learning on developing trading algorithms with Python.
- Kaggle: An excellent platform for practicing data science and machine learning, with numerous datasets and competitions related to financial markets.
Conclusion
The volatile nature of cryptocurrency markets demands robust and adaptive trading strategies. Python, with its simplicity and powerful libraries, offers an ideal platform for developing and executing these strategies. By leveraging Python, traders can analyze vast amounts of data, create sophisticated algorithms, and manage risks effectively. As the cryptocurrency market continues to evolve, staying informed and continuously learning will be key to success.