File Handling in Python: A Comprehensive Guide
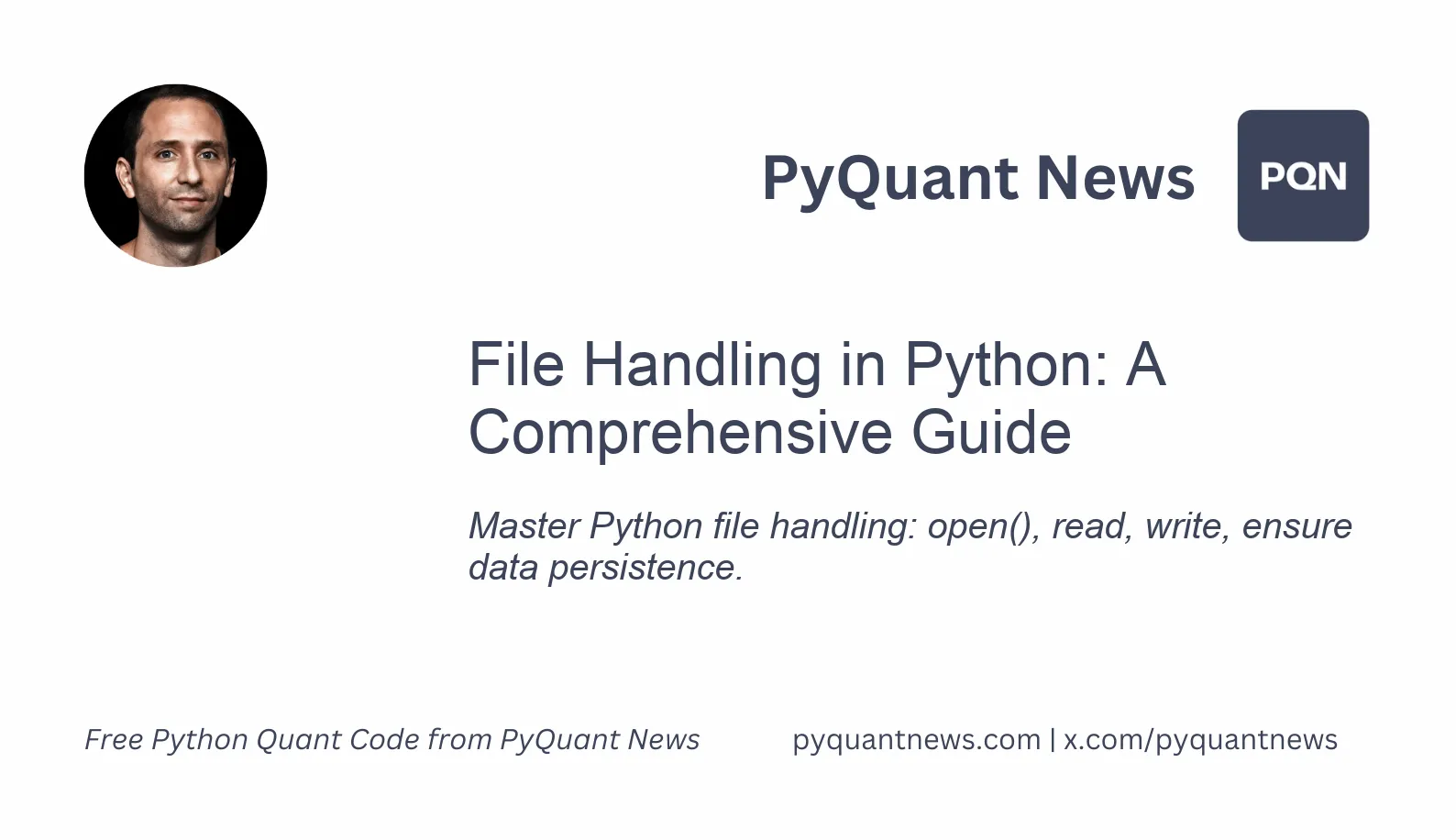
File Handling in Python: A Comprehensive Guide
In the world of programming, ensuring data persistence and efficient data manipulation is key to developing robust software. Python, known for its simplicity and versatility, offers powerful features for file handling that every developer should master.
Introduction
Data persistence means that data lasts beyond the runtime of a program. This is crucial for applications that need to save information between sessions, such as databases, configuration files, or user-generated content. Python provides straightforward methods to read from and write to files, making it a top choice for developers focusing on data persistence.
Why File Handling in Python Matters
File handling in Python is fundamental for creating, reading, updating, and deleting files. Python's built-in functions and modules simplify these tasks, making data management easier. The ability to manipulate files is essential for:
- Data Storage: Saving user preferences, configurations, and state information.
- Data Processing: Reading large datasets for analysis, transformation, and visualization.
- Inter-Process Communication: Writing data that can be read by other programs or processes.
Basics of File Handling in Python
Opening and Closing Files
The first step in file handling is opening a file. Python's open()
function is used for this purpose. It requires two arguments: the file path and the mode in which the file should be opened.
file = open('example.txt', 'r') # 'r' stands for read mode
Modes include:
'r'
: Read (default)'w'
: Write (creates a new file if it doesn't exist or truncates the file if it exists)'a'
: Append (creates a new file if it doesn't exist)'b'
: Binary mode (e.g.,'rb'
or'wb'
)
Closing a file is important to free up system resources and avoid potential data corruption. This is done using the close()
method.
file.close()
Reading from Files
Reading data from a file can be done in several ways:
Reading the Entire File
The read()
method reads the entire file content as a string.
file = open('example.txt', 'r')
content = file.read()
print(content)
file.close()
Reading Line by Line
The readline()
method reads one line at a time, which is useful for large files.
file = open('example.txt', 'r')
line = file.readline()
while line:
print(line)
line = file.readline()
file.close()
Reading All Lines
The readlines()
method reads all lines into a list.
file = open('example.txt', 'r')
lines = file.readlines()
for line in lines:
print(line)
file.close()
Writing to Files
Writing data to a file is straightforward. Using the write()
method, you can write strings to a file.
file = open('example.txt', 'w')
file.write('Hello, World!')
file.close()
For appending data, use the 'a'
mode.
file = open('example.txt', 'a')
file.write('\nAppending a new line.')
file.close()
Using the with
Statement
Python’s with
statement simplifies file handling by ensuring that files are automatically closed after the block of code is executed, even in case of exceptions.
with open('example.txt', 'r') as file:
content = file.read()
print(content)
This approach is preferred as it ensures that the file is properly closed, even if an exception occurs.
Advanced File Handling Techniques
Working with Binary Files
Binary files store data in a format that is not human-readable, such as images, audio files, or compiled programs. Reading and writing to these files involves using the 'b'
mode.
# Writing to a binary file
with open('example.bin', 'wb') as file:
file.write(b'\x00\xFF\x00\xFF')
# Reading from a binary file
with open('example.bin', 'rb') as file:
data = file.read()
print(data)
File Positioning
Python allows you to manipulate the file pointer using the seek()
and tell()
methods. The seek()
method moves the file pointer to a specified location, and the tell()
method returns the current position.
with open('example.txt', 'r') as file:
file.seek(10)
print(file.read())
print(file.tell())
Working with CSV Files
CSV (Comma-Separated Values) files are widely used for storing tabular data. Python's csv
module provides functionality to read from and write to CSV files.
import csv
# Writing to a CSV file
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age', 'City'])
writer.writerow(['Alice', 30, 'New York'])
writer.writerow(['Bob', 25, 'San Francisco'])
# Reading from a CSV file
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
JSON Files
JSON (JavaScript Object Notation) is a popular data format for exchanging data between a server and a web application. Python's json
module allows you to work with JSON data.
import json
# Writing to a JSON file
data = {'name': 'Alice', 'age': 30, 'city': 'New York'}
with open('example.json', 'w') as file:
json.dump(data, file)
# Reading from a JSON file
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
Error Handling in File Operations
Handling errors is important to ensure that your program behaves correctly under unexpected conditions, preventing crashes and providing meaningful feedback to the user. Python provides the try
and except
blocks to catch and handle exceptions.
try:
with open('non_existent_file.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print('The file does not exist.')
Best Practices for File Handling
To ensure robust and efficient file handling in your Python projects, consider the following best practices:
- Use Context Managers: Always use the
with
statement to ensure that files are properly closed. - Handle Exceptions: Implement error handling to manage unexpected scenarios.
- Check File Existence: Use
os.path.exists()
to check if a file exists before performing operations. - Use Absolute Paths: Avoid relative paths to prevent issues related to the current working directory.
Resources for Further Learning
To deepen your understanding of file handling in Python, consider exploring the following resources:
- Python Official Documentation: A comprehensive guide to Python's built-in functions, including file handling.
- Automate the Boring Stuff with Python by Al Sweigart: A practical book that covers file handling and other essential Python concepts.
- Real Python: A website offering tutorials and articles on various Python topics, including file handling.
- Python for Data Analysis by Wes McKinney: A book that covers data manipulation and analysis using Python.
- Python Crash Course by Eric Matthes: A beginner-friendly book that includes chapters on file handling and data persistence.
Conclusion
Mastering file handling in Python is fundamental for building robust and efficient applications. By understanding how to read from and write to files, you ensure data persistence and effective manipulation. Utilize Python's simplicity and extensive libraries, follow best practices, and delve into recommended resources to further enhance your skills. With these tools, you're well-equipped to handle any file handling challenges in your Python projects. Happy coding!