Event-Driven Backtesting for Trading Strategies
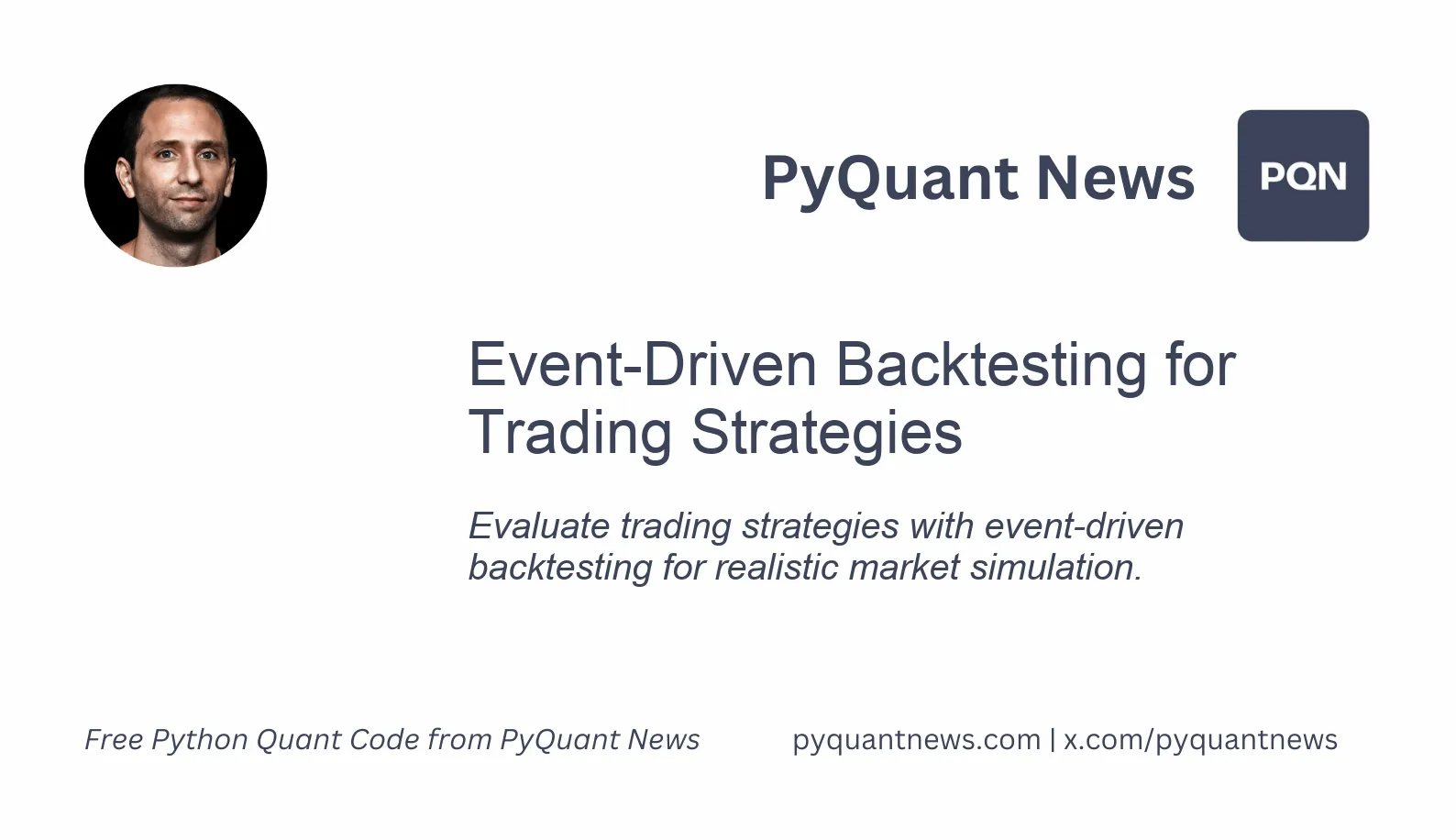
Event-Driven Backtesting for Trading Strategies
In the high-stakes world of financial trading, accurately predicting market movements can mean the difference between success and failure. Imagine a trader simulating their strategy during events like the 2008 financial crisis or the 2020 pandemic. This is where event-driven backtesting frameworks become invaluable for trading strategy evaluation.
Understanding Event-Driven Backtesting
What is Event-Driven Backtesting?
Unlike traditional backtesting, which relies on fixed time intervals, event-driven backtesting responds to specific market events. These events can range from price changes and news releases to earnings reports and economic indicators. By focusing on these events, event-driven frameworks more closely reflect real trading scenarios, making them ideal for evaluating trading strategies.
Why Use Event-Driven Backtesting?
Realistic Simulation
Traders often react to events rather than fixed time intervals. Event-driven backtesting simulates real-world trading conditions more accurately, making it a closer proxy to actual trading.
Complex Strategy Evaluation
Sophisticated trading strategies often rely on complex triggers and conditions. Event-driven frameworks can handle these intricacies more effectively than traditional methods, providing a more realistic simulation.
Handling Latency and Slippage
Event-driven backtesting can account for market latency and slippage, factors that significantly impact high-frequency trading strategies.
Key Components of an Event-Driven Backtesting Framework
Implementing an event-driven backtesting framework requires understanding its core components. Here are the essential elements:
Event Queue
The event queue is the backbone of the framework. It stores and manages all events, ensuring they are processed in the correct order. Events can include market data updates, execution reports, and custom user-defined events.
Event Handler
The event handler processes events from the queue and determines the appropriate action. This could involve updating market data, executing trades, or recalculating strategy parameters.
Execution Engine
The execution engine simulates the actual trading environment. It processes trade orders generated by the event handler and provides feedback on order execution, including fills, partial fills, and rejections.
Portfolio Manager
The portfolio manager maintains the state of the trading portfolio. It tracks positions, account balances, and performance metrics. It ensures that the strategy adheres to risk management rules and constraints.
Data Handler
The data handler provides access to historical and real-time market data. It ensures data integrity and consistency, allowing the framework to simulate market conditions accurately.
Implementing an Event-Driven Backtesting Framework
Define Events and Triggers
The first step is defining the events and triggers that will drive the strategy. These can include market data updates, news releases, or custom indicators. Each event should have a clear definition and associated triggers.
Build the Event Queue
The event queue is a priority queue that sorts events based on their timestamps. This ensures that events are processed in the correct order.
Develop the Event Handler
The event handler processes events from the queue and triggers the appropriate actions. This component should be flexible and extensible, allowing for the implementation of complex trading strategies.
Integrate the Execution Engine
The execution engine simulates the actual trading environment, processing trade orders and providing feedback on order execution. It should account for market latency and slippage, ensuring that the backtest results are realistic.
Implement the Portfolio Manager
The portfolio manager maintains the state of the trading portfolio, tracking positions, account balances, and performance metrics. It should enforce risk management rules and constraints.
Set Up the Data Handler
The data handler provides access to historical and real-time market data. It should ensure data integrity and consistency, allowing the framework to simulate market conditions accurately.
Test and Validate
Once the framework is implemented, it is essential to test and validate its functionality. This involves running the framework on historical data and comparing the results to known outcomes. Any discrepancies should be investigated and resolved.
Benefits of Event-Driven Backtesting
Enhanced Realism
Event-driven backtesting provides a more realistic simulation of real-world trading conditions. By responding to discrete events, the framework can mimic the behavior of actual traders, providing more accurate performance metrics.
Flexibility and Extensibility
Event-driven frameworks are highly flexible and extensible. They can accommodate a wide range of trading strategies, from simple moving average crossovers to complex machine learning models.
Improved Risk Management
By incorporating risk management rules and constraints, event-driven backtesting frameworks can help traders manage risk more effectively. This is particularly important for high-frequency trading strategies.
Better Handling of Market Latency and Slippage
Event-driven frameworks can account for market latency and slippage, factors that often impact the performance of trading strategies. By simulating these factors, the framework can provide more accurate performance metrics.
Challenges and Considerations
Data Quality and Integrity
The accuracy of backtesting results depends heavily on the quality and integrity of the data used. It is essential to ensure that historical and real-time market data is accurate and consistent.
Computational Complexity
Event-driven backtesting frameworks can be computationally intensive, particularly for high-frequency trading strategies. It is essential to optimize the framework for performance, ensuring that it can handle large volumes of data and events efficiently.
Debugging and Validation
Debugging and validating event-driven frameworks can be challenging due to their complexity. It is essential to implement robust testing and validation procedures to ensure that the framework operates correctly and produces accurate results.
Case Study: Implementing an Event-Driven Framework in Python
To illustrate the implementation of an event-driven backtesting framework, let's consider a case study using Python, a popular programming language for financial applications.
Step 1: Define Events and Triggers
In this case study, we will define two types of events: market data updates and trade executions. Market data updates trigger the strategy to evaluate trading signals, while trade executions update the portfolio state.
class MarketEvent:
def __init__(self, timestamp, symbol, price):
self.timestamp = timestamp
self.symbol = symbol
self.price = price
class TradeEvent:
def __init__(self, timestamp, symbol, quantity, price):
self.timestamp = timestamp
self.symbol = symbol
self.quantity = quantity
self.price = price
Step 2: Build the Event Queue
We will use Python's queue.PriorityQueue
to implement the event queue, ensuring that events are processed in the correct order.
import queue
event_queue = queue.PriorityQueue()
Step 3: Develop the Event Handler
The event handler will process events from the queue and trigger the appropriate actions. In this case, it will evaluate trading signals and generate trade orders.
class EventHandler:
def __init__(self, strategy, portfolio_manager):
self.strategy = strategy
self.portfolio_manager = portfolio_manager
def handle_event(self, event):
if isinstance(event, MarketEvent):
signals = self.strategy.evaluate(event)
for signal in signals:
order = self.portfolio_manager.generate_order(signal)
event_queue.put(order)
elif isinstance(event, TradeEvent):
self.portfolio_manager.update(event)
Step 4: Integrate the Execution Engine
The execution engine will simulate the actual trading environment, processing trade orders and providing feedback on order execution.
class ExecutionEngine:
def execute_order(self, order):
# Simulate order execution
trade_event = TradeEvent(order.timestamp, order.symbol, order.quantity, order.price)
event_queue.put(trade_event)
Step 5: Implement the Portfolio Manager
The portfolio manager will maintain the state of the trading portfolio, tracking positions, account balances, and performance metrics.
class PortfolioManager:
def __init__(self):
self.positions = {}
self.balance = 100000 # Initial balance
def generate_order(self, signal):
# Generate trade order based on signal
pass
def update(self, trade_event):
# Update portfolio state based on trade execution
pass
Step 6: Set Up the Data Handler
The data handler will provide access to historical and real-time market data, ensuring data integrity and consistency.
class DataHandler:
def __init__(self, data):
self.data = data
def get_next_event(self):
# Retrieve the next market event from the data
pass
Step 7: Test and Validate
Finally, we will test and validate the framework by running it on historical data and comparing the results to known outcomes.
data_handler = DataHandler(historical_data)
event_handler = EventHandler(strategy, portfolio_manager)
execution_engine = ExecutionEngine()
while not event_queue.empty():
event = event_queue.get()
event_handler.handle_event(event)
if isinstance(event, MarketEvent):
next_event = data_handler.get_next_event()
if next_event:
event_queue.put(next_event)
Recommended Resources
For readers interested in diving deeper into the world of event-driven backtesting frameworks, here are some valuable resources:
"Python for Finance: Analyze Big Financial Data" by Yves Hilpisch
This comprehensive book covers various aspects of financial data analysis using Python, including backtesting and algorithmic trading.
QuantConnect
QuantConnect is an open-source algorithmic trading platform that provides a robust event-driven backtesting framework. It offers extensive documentation and tutorials to help traders get started.
Zipline
Zipline is a Pythonic algorithmic trading library that provides an event-driven backtesting framework. It is widely used by the Quantopian community and offers extensive documentation and examples.
"Algorithmic Trading: Winning Strategies and Their Rationale" by Ernest P. Chan
This book provides insights into algorithmic trading strategies and includes practical examples of backtesting using event-driven frameworks.
QuantStart
QuantStart is a valuable online resource for quantitative trading and backtesting. It offers articles, tutorials, and courses on implementing event-driven backtesting frameworks and developing trading strategies.
Conclusion
Event-driven backtesting frameworks represent a significant advancement in trading strategy evaluation. By focusing on discrete events and mimicking real-world trading conditions, these frameworks provide a more realistic and flexible approach to backtesting.
In summary, event-driven backtesting frameworks offer a realistic, flexible, and accurate method for evaluating trading strategies. By focusing on discrete events, these frameworks can better simulate real-world conditions, making them invaluable tools for traders.
Whether you're a seasoned trader or new to algorithmic trading, consider integrating event-driven backtesting frameworks into your strategy evaluation process. The investment in time and resources can pay significant dividends in your trading performance.