Event-Driven Architecture in Python for Trading
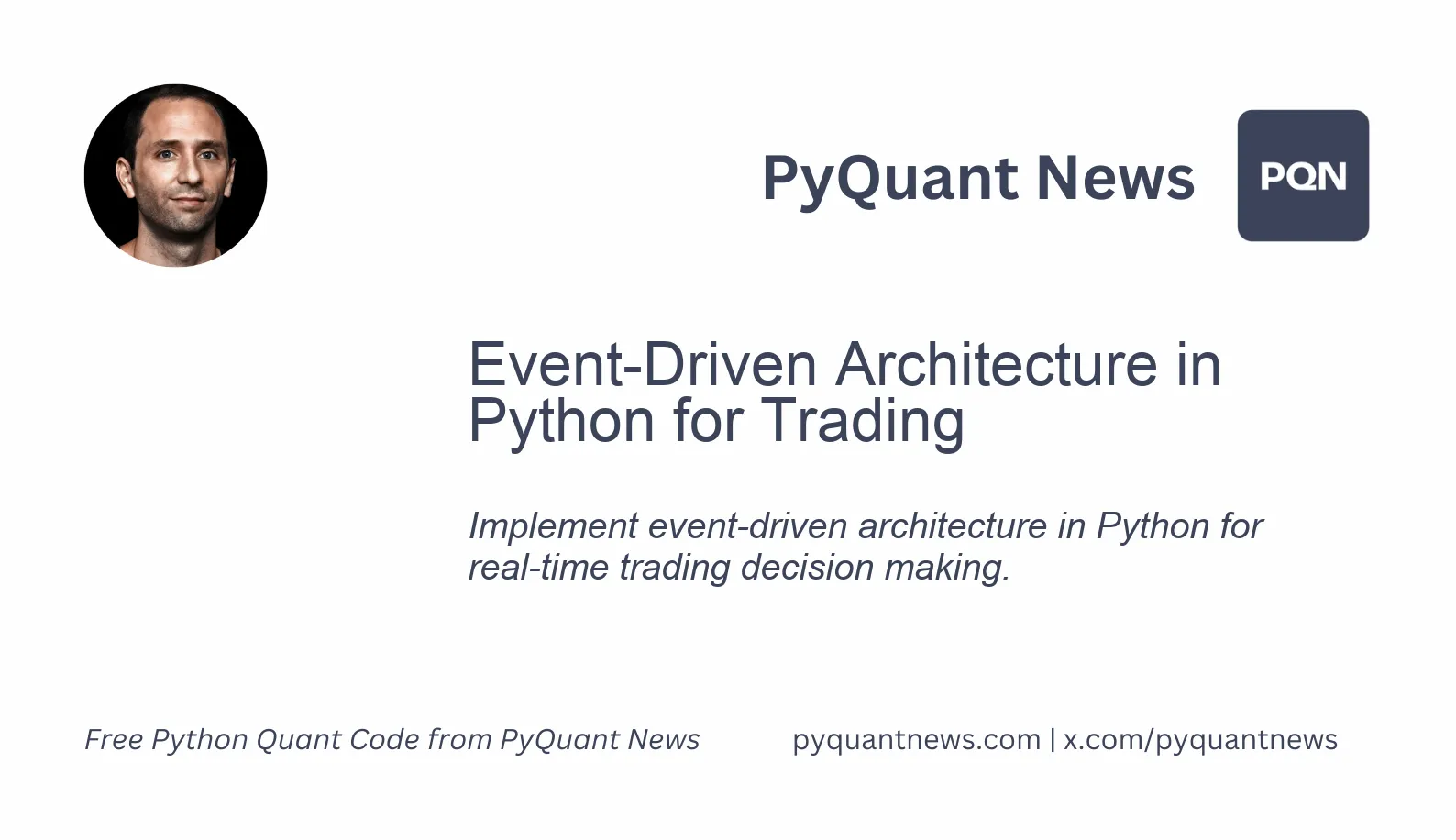
Event-Driven Architecture in Python for Trading
In the fast-paced realm of financial markets, making quick and informed decisions is crucial. The rise of advanced computing and sophisticated algorithms has made real-time trading an integral part of modern finance. One effective method gaining popularity is implementing event-driven architecture (EDA) using Python. This approach allows for rapid trading decision-making by reacting to market events as they occur. This article delves into the basics of EDA, the advantages of using Python, a step-by-step guide to building an EDA-based trading system, and considerations to keep in mind.
Understanding Event-Driven Architecture
Event-driven architecture is a design paradigm where the program's flow is determined by events such as user actions, sensor outputs, or message passing. In trading, events can include market data updates, trade executions, or changes in strategy parameters. The core principle is to react to these events in real-time, enabling swift decisions based on the latest information.
Key Components of EDA
- Event Producers: Generate events like market data feeds, news sources, or external APIs.
- Event Consumers: Process the events, including trading algorithms, risk management systems, or alert mechanisms.
- Event Channels: Transmit events from producers to consumers, commonly using messaging systems like Kafka or RabbitMQ.
- Event Processors: Handle the logic for processing events, such as filters, transformers, and aggregators.
Why Python?
Python is highly favored for implementing EDA in trading due to its simplicity, extensive libraries, and robust community support. Here’s why Python stands out:
- Ease of Use: Python’s clean and readable syntax makes it accessible for developers at all levels.
- Comprehensive Libraries: Libraries like
pandas
for data manipulation,numpy
for numerical operations, andscikit-learn
for machine learning make Python versatile. - Integration Capabilities: Python seamlessly integrates with other languages and systems, facilitating smooth communication between different components.
- Community and Support: A large, active community provides a wealth of resources and support for developers working on trading systems.
Implementing EDA in Python for Real-Time Trading
To build an event-driven trading system in Python, follow these key steps:
Setting Up the Environment
Before writing the code, set up the development environment by installing necessary libraries and creating a virtual environment.
# Create a virtual environment
python3 -m venv trading_env
source trading_env/bin/activate
# Install required libraries
pip install pandas numpy scikit-learn kafka-python
Defining the Event Model
Defining an event model standardizes the format of the data to be processed, ensuring consistency and reliability. In trading, an event might contain information such as the symbol, price, volume, and timestamp.
from dataclasses import dataclass
from datetime import datetime
@dataclass
class MarketEvent:
symbol: str
price: float
volume: int
timestamp: datetime
Setting Up Event Producers
Event producers generate market events. For example, simulate market data using a simple generator function.
import random
from datetime import datetime
def market_data_generator(symbols, num_events):
for _ in range(num_events):
event = MarketEvent(
symbol=random.choice(symbols),
price=round(random.uniform(100, 200), 2),
volume=random.randint(1, 1000),
timestamp=datetime.now()
)
yield event
symbols = ['AAPL', 'GOOGL', 'MSFT']
events = market_data_generator(symbols, 100)
Setting Up Event Channels
For real-time event transmission, use a messaging system like Kafka. Set up a Kafka producer to send market events.
from kafka import KafkaProducer
import json
producer = KafkaProducer(
bootstrap_servers='localhost:9092',
value_serializer=lambda v: json.dumps(v.__dict__).encode('utf-8')
)
for event in events:
producer.send('market_events', event)
Setting Up Event Consumers
Event consumers process the events received from the event channel. Set up a Kafka consumer to receive and process market events.
from kafka import KafkaConsumer
consumer = KafkaConsumer(
'market_events',
bootstrap_servers='localhost:9092',
value_deserializer=lambda m: MarketEvent(**json.loads(m.decode('utf-8')))
)
for message in consumer:
event = message.value
print(f"Received event: {event.symbol} at {event.price} with volume {event.volume}")
Implementing Event Processors
Event processors handle the logic for processing events. For instance, a simple trading strategy might buy a stock if the price falls below a certain threshold.
class SimpleTradingStrategy:
def __init__(self, threshold):
self.threshold = threshold
def process_event(self, event):
if event.price < self.threshold:
print(f"Buying {event.symbol} at {event.price}")
strategy = SimpleTradingStrategy(threshold=150)
for message in consumer:
event = message.value
strategy.process_event(event)
Challenges and Considerations
Implementing an event-driven trading system involves challenges. Here are some considerations:
- Latency: Minimizing latency is vital. Optimize the event processing pipeline and ensure efficient communication between components. In-memory data stores like Redis can speed up data access.
- Scalability: The system should scale horizontally as the event volume increases. Cloud-based solutions offer auto-scaling features that can be beneficial.
- Fault Tolerance: Ensure the system is resilient to failures by implementing redundancy and failover mechanisms. Multiple Kafka brokers can help.
- Security: Protect the system from unauthorized access and ensure data integrity using secure communication channels, authentication, and encryption.
Resources for Further Learning
To further understand EDA and its application in trading, explore these resources:
- "Designing Data-Intensive Applications" by Martin Kleppmann: A comprehensive overview of data-driven architectures, including event-driven systems.
- "Algorithmic Trading and DMA" by Barry Johnson: Insights into algorithmic trading, covering strategies and the technical infrastructure for real-time trading.
- Kafka: The Definitive Guide by Neha Narkhede, Gwen Shapira, and Todd Palino: Essential for understanding Kafka, a powerful messaging system used in event-driven architectures.
- Coursera’s “Machine Learning for Trading” by Georgia Tech: Covers the application of machine learning techniques in trading, providing practical insights and hands-on experience.
- PyCon Talks: Annual conference for Python enthusiasts, offering many online talks on event-driven programming and real-time data processing.
Conclusion
Implementing event-driven architecture in Python for real-time trading decision-making offers a powerful approach to navigating the volatile world of financial markets. Python's simplicity and robust ecosystem make it an ideal choice for building systems that react to market events swiftly and accurately. While addressing challenges like latency, scalability, and security is necessary, the benefits of EDA in providing timely and informed trading decisions are undeniable. As financial markets evolve, event-driven architecture will continue to play a leading role in shaping the tools and techniques that drive them.