Building Basic Trading Algorithms with Python
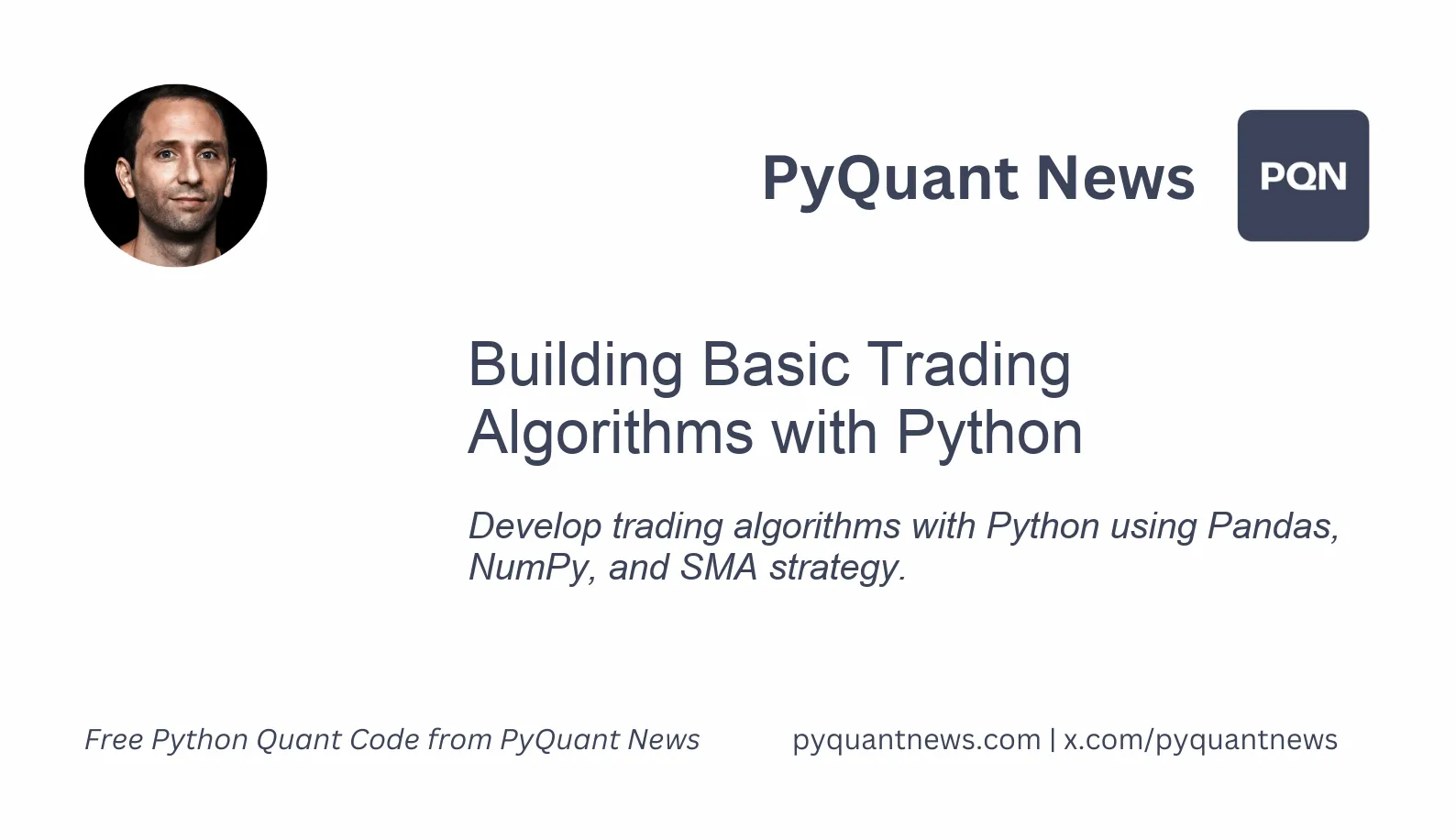
Building Basic Trading Algorithms with Python
In the fast-paced world of finance, algorithmic trading has revolutionized how traders operate. Automating trading strategies, analyzing vast datasets in real-time, and executing trades with precision are game-changers. Python, with its simplicity and rich ecosystem, has become the go-to language for developing these algorithms. This article explores creating basic trading algorithms with Python, focusing on data manipulation with Pandas and numerical computations with NumPy.
Introduction to Algorithmic Trading
Algorithmic trading, or algo-trading, uses computer programs to execute trading strategies based on predefined criteria. These algorithms process large datasets, identify trading opportunities, and execute trades faster than any human. Benefits include improved accuracy, reduced emotional bias, and the ability to backtest strategies on historical stock price data.
Python's ease of use and extensive library support make it ideal for algorithmic trading. Pandas and NumPy are particularly powerful for data manipulation and numerical computations, respectively.
Setting Up Your Environment
Before developing an algorithm, set up your Python environment. Follow these steps:
- Install Python: First, ensure Python is installed on your system. Download it from the official Python website.
- Install Pandas and NumPy: Use pip, Python's package installer, to install these libraries. Open your terminal or command prompt and run the following commands:
pip install pandas
pip install numpy- Jupyter Notebook: For an interactive coding experience, consider using Jupyter Notebook. It allows you to write and execute code in a web-based environment. To install it, use the following command:
pip install notebook
Basic Concepts of Pandas and NumPy
Before diving into building trading algorithms, it’s important to grasp the basics of Pandas and NumPy.
Pandas
Pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames and Series, essential for handling time-series data in trading.
- DataFrame: A 2-dimensional labeled data structure with columns of different types. Think of it as an in-memory 2D table.
- Series: A 1-dimensional labeled array capable of holding any data type.
NumPy
NumPy is the fundamental package for numerical computing in Python. It supports arrays, matrices, and a variety of mathematical functions to operate on these data structures.
- Array: A grid of values, all of the same type, indexed by a tuple of non-negative integers.
Developing a Simple Moving Average (SMA) Trading Algorithm
One of the simplest trading strategies is based on the Simple Moving Average (SMA). The SMA is calculated by averaging a set of prices over a specific period. It's commonly used to identify trends and potential buy/sell signals.
Step-by-Step Implementation
- Import Libraries:
import pandas as pd # Importing Pandas for data manipulation
import numpy as np # Importing NumPy for numerical operations
import matplotlib.pyplot as plt # Importing Matplotlib for data visualization- Load Data: For this example, we'll use historical stock price data from a CSV file. Ensure you have a file named
stock_data.csv
with columns such asDate
andClose
. data = pd.read_csv('stock_data.csv') # Load the data
data['Date'] = pd.to_datetime(data['Date']) # Convert Date column to datetime
data.set_index('Date', inplace=True) # Set Date as index- Calculate SMA: We'll calculate two SMAs: a short-term and a long-term SMA.
data['SMA_20'] = data['Close'].rolling(window=20).mean() # 20-day SMA
data['SMA_50'] = data['Close'].rolling(window=50).mean() # 50-day SMA- Generate Buy/Sell Signals: We'll create a straightforward strategy: buy when the short-term SMA crosses above the long-term SMA, and sell when it crosses below.
data['Signal'] = 0 # Initialize the Signal column
data['Signal'][20:] = np.where(data['SMA_20'][20:] > data['SMA_50'][20:], 1, 0) # Buy signal
data['Position'] = data['Signal'].diff() # Position is the difference in signals- Visualize the Data: Plot the data to see the stock price along with the SMAs and trading signals.
plt.figure(figsize=(12,8))
plt.plot(data['Close'], label='Close Price', alpha=0.5)
plt.plot(data['SMA_20'], label='20-Day SMA', alpha=0.75)
plt.plot(data['SMA_50'], label='50-Day SMA', alpha=0.75)
plt.scatter(data.index, data['Position'] == 1, label='Buy Signal', marker='^', color='green')
plt.scatter(data.index, data['Position'] == -1, label='Sell Signal', marker='v', color='red')
plt.title('Stock Price with Buy/Sell Signals')
plt.xlabel('Date')
plt.ylabel('Price')
plt.legend()
plt.show()
This simple algorithm provides a basic framework for a trading strategy based on moving averages. While it's not a guaranteed path to profits, it serves as an excellent starting point for understanding how trading algorithms work.
Enhancing the Algorithm
The basic SMA strategy can be improved in several ways to enhance its performance and robustness.
- Optimize Parameters: Experiment with different window sizes for the moving averages to find the optimal combination.
- Add Indicators: Incorporate other technical indicators like Relative Strength Index (RSI) or Bollinger Bands to refine buy/sell signals.
- Risk Management: Implement risk management techniques such as stop-loss and take-profit levels to protect against significant losses.
Backtesting the Algorithm
Backtesting is a critical step in developing trading algorithms. It involves testing the strategy on historical stock price data to evaluate its performance before deploying it in live trading.
Implementing Backtesting
- Calculate Returns:
data['Daily_Return'] = data['Close'].pct_change() # Calculate daily returns
data['Strategy_Return'] = data['Daily_Return'] * data['Position'].shift(1) # Strategy returns- Compute Cumulative Returns:
data['Cumulative_Market_Return'] = (1 + data['Daily_Return']).cumprod() # Market cumulative returns
data['Cumulative_Strategy_Return'] = (1 + data['Strategy_Return']).cumprod() # Strategy cumulative returns- Plot Cumulative Returns:
plt.figure(figsize=(12,8))
plt.plot(data['Cumulative_Market_Return'], label='Market Return', alpha=0.75)
plt.plot(data['Cumulative_Strategy_Return'], label='Strategy Return', alpha=0.75)
plt.title('Market vs. Strategy Returns')
plt.xlabel('Date')
plt.ylabel('Cumulative Return')
plt.legend()
plt.show()
Backtesting results offer valuable insights into the strategy's performance, helping to identify strengths and weaknesses. It’s crucial to backtest on multiple datasets and timeframes to ensure robustness.
Resources for Further Learning
Developing and refining trading algorithms is a continuous learning process. Here are some resources to help you deepen your knowledge:
- Quantitative Finance with Python: A Practical Guide by Chris Kelliher: This book provides a comprehensive introduction to quantitative finance using Python, covering a range of topics from basic concepts to advanced strategies.
- Algorithmic Trading and Quantitative Strategies by Coursera: An online course that covers the fundamentals of algorithmic trading, including strategy development, backtesting, and risk management.
- Pandas Documentation: The official documentation for the Pandas library is an invaluable resource for understanding its vast array of functionalities and how to apply them to your data.
- NumPy Documentation: The official documentation for NumPy provides detailed information on its array operations, mathematical functions, and more.
- Kaggle: A platform for data science competitions and datasets. Kaggle offers a wealth of resources, including tutorials, code snippets, and discussions on trading algorithms.
Conclusion
Developing trading algorithms using Python, Pandas, and NumPy is a powerful method to leverage data and execute strategies with precision. While we’ve covered the basics of a Simple Moving Average strategy, the possibilities are endless. By understanding the fundamentals and utilizing the resources mentioned, you can embark on a journey to becoming proficient in algorithmic trading, potentially unlocking new opportunities in the financial markets. Happy coding and trading!