Build a Stock Database Locally with Polars
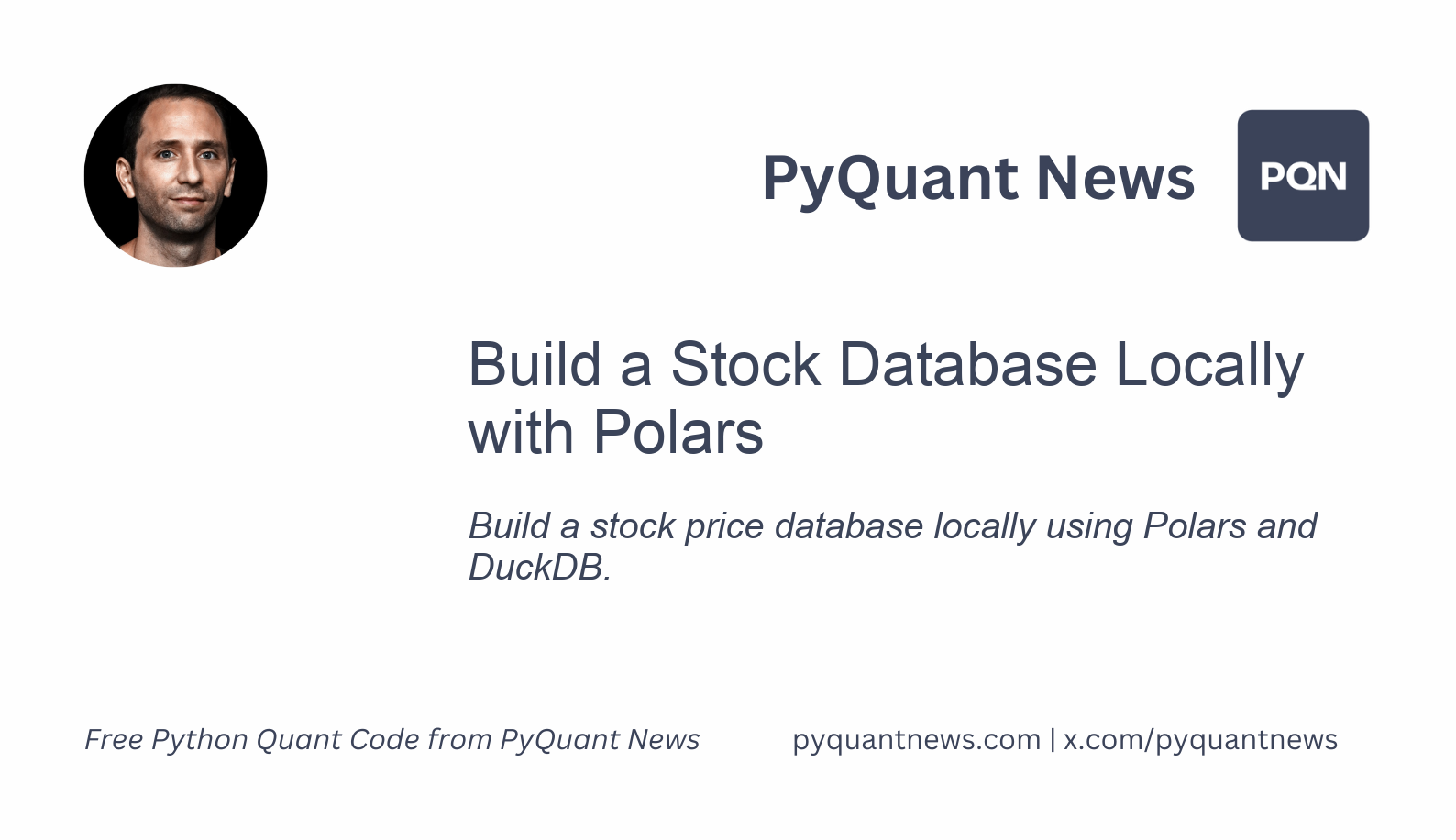
Build a Stock Database Locally with Polars
In the finance world, efficient finance data management is crucial for analysts and traders who rely heavily on timely stock price data. While cloud services have traditionally handled these tasks, the advent of Polars DataFrame and DuckDB SQL enables powerful local data processing. This article guides you through setting up a local stock price database using these tools.
Introduction to Polars and DuckDB
Before we dive into building a stock price database, let's explore these two key libraries.
Polars is a Rust and Python DataFrame library renowned for its speed and efficiency in data manipulation. It provides a modern alternative to Pandas, offering swift handling of large datasets. Polars DataFrame allows complex data transformations with an intuitive API, making it ideal for finance data management.
DuckDB is an in-process SQL OLAP database management system, often dubbed the SQLite of analytics. It excels in querying large datasets with its columnar format and vectorized engine processing. DuckDB SQL integrates seamlessly with various data formats, including Polars DataFrames, making it versatile for data analysis.
Setting Up the Environment
To build a stock database locally, begin by setting up your environment. Install Polars and DuckDB along with their dependencies.
Installation Process
Use Python's package installer, pip, to install Polars and DuckDB. Open your terminal and execute the following commands:
pip install polars
pip install duckdb
Verify each installation by importing the libraries in Python:
python -c "import polars"
python -c "import duckdb"
These steps prepare your system for local data processing and financial data analysis.
Collecting Stock Price Data
Next, gather historical stock prices. You can source this data from APIs like Alpha Vantage, Yahoo Finance, or IEX Cloud. For this guide, assume you have a CSV file with historical stock data. If not, consider looking up tutorials for downloading data from Yahoo Finance.
Constructing the Stock Price Database
With Polars and DuckDB installed, and stock data in hand, you can now build your stock price database. This process involves data loading, transformation using Polars, and storing in DuckDB for further analysis.
Data Loading and Transformation with Polars
Polars offers a robust API for loading and transforming data efficiently. Begin by importing the necessary packages and loading your CSV into a Polars DataFrame:
import polars as pl
df = pl.read_csv('historical_stock_prices.csv')
Filter this data for specific criteria, such as ticker symbols or date ranges:
filtered_df = df.filter(
(pl.col('ticker') == 'AAPL') &
(pl.col('date') >= '2022-01-01') &
(pl.col('date') <= '2022-12-31')
)
Polars DataFrame enables complex operations with minimal code, allowing you to create new columns, apply aggregations, or join DataFrames with ease.
Storing Data with DuckDB
After transforming data with Polars, store it in DuckDB for effective querying. DuckDB SQL interacts directly with Polars DataFrames. Establish a DuckDB connection and create a database file:
import duckdb
con = duckdb.connect(database='stock_prices.duckdb', read_only=False)
Write the filtered Polars DataFrame to a DuckDB table:
con.execute("CREATE TABLE IF NOT EXISTS stock_prices AS SELECT * FROM filtered_df")
With data stored in DuckDB, leverage SQL queries for comprehensive data analysis. DuckDB SQL supports various operations, including aggregation, filtering, and joining data for deeper insights.
Utilizing SQL for Analysis
DuckDB SQL allows sophisticated data analysis. Here are some SQL examples:
Calculating Moving Averages
To identify trends, calculate moving averages of stock prices:
SELECT
date,
AVG(close) OVER (ORDER BY date ROWS BETWEEN 4 PRECEDING AND CURRENT ROW) AS moving_average
FROM
stock_prices
WHERE
ticker = 'AAPL'
This SQL query calculates a 5-day moving average for Apple's stock prices.
Detecting Price Breakouts
Identify potential price breakouts by finding days where closing prices exceed a set threshold:
SELECT
date,
close
FROM
stock_prices
WHERE
ticker = 'AAPL' AND close > 150
ORDER BY
date
This returns dates when Apple's closing price surpassed $150.
Benefits of Polars and DuckDB
Utilizing Polars DataFrame and DuckDB SQL for building a local stock price database offers numerous advantages:
- Speed and Efficiency: The Rust-based Polars and DuckDB's vectorized engine provide fast processing for large datasets.
- User-Friendly: Both tools have intuitive APIs for straightforward data loading, transformation, and analysis.
- Local Control: By building a local database, you maintain control over your data, minimizing reliance on external services.
- Scalability: Handle larger datasets than traditional tools like Pandas, supporting scalable analysis.
- SQL Integration: DuckDB SQL enables powerful analysis using a familiar language.
Conclusion
As the volume of financial data grows, efficient management becomes increasingly important. By building a stock price database locally with Polars DataFrame and DuckDB SQL, you can achieve fast, scalable data processing without relying on cloud services. Whether you're an analyst or a data enthusiast, mastering these tools can unlock new insights from your stock data. This guide equips you to harness the full potential of your financial data.