Basic Trading Algorithms in Python
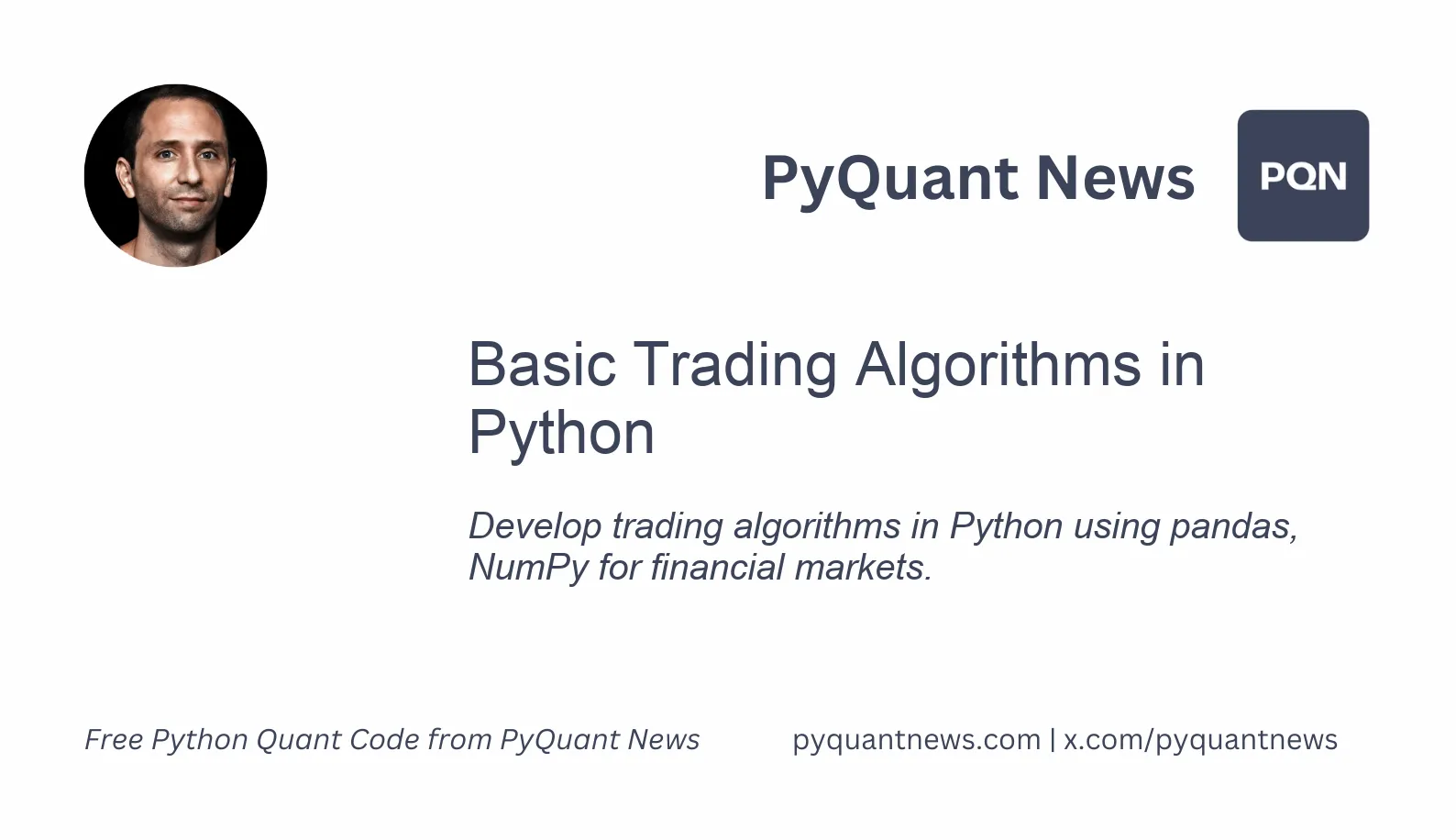
Basic Trading Algorithms in Python
The rise of algorithmic trading has transformed financial markets, allowing traders to make precise, data-driven decisions. Python, with its powerful libraries like pandas and NumPy, is a top choice for developing trading algorithms. This article will guide you through creating basic trading algorithms in Python, making use of these libraries.
Introduction to Algorithmic Trading
Algorithmic trading uses computer programs to perform trades at speeds and frequencies beyond human capability. These algorithms analyze market data, spot trading opportunities, and execute orders based on predefined criteria. The key benefits include:
- Speed: Orders are executed in milliseconds.
- Accuracy: Reduces human error.
- Consistency: Operates continuously without fatigue.
Why Use Python for Trading Algorithms?
Python's simplicity and versatility make it ideal for trading algorithms. This article focuses on two primary libraries:
- pandas: For data manipulation and analysis, providing structures and functions to work with structured data effortlessly.
- NumPy: For numerical computing, handling large, multi-dimensional arrays and matrices along with a suite of mathematical functions.
Other useful Python libraries include:
- scikit-learn: For machine learning.
- matplotlib: For data visualization.
Setting Up the Environment
Before we begin coding, ensure you have Python along with the necessary libraries installed. Install pandas and NumPy using pip:
pip install pandas
pip install numpy
Data Acquisition and Preparation
The first step in developing trading algorithms is acquiring and preparing the data. We'll use historical stock price data from Yahoo Finance, fetched using the pandas_datareader
library.
import pandas_datareader.data as web
import datetime
# Define the start and end date
start = datetime.datetime(2020, 1, 1)
end = datetime.datetime(2022, 1, 1)
# Fetch data for a particular stock
stock_data = web.DataReader('AAPL', 'yahoo', start, end)
print(stock_data.head())
This data includes columns like 'High', 'Low', 'Open', 'Close', 'Volume', and 'Adj Close'. We will focus on 'Adj Close' prices.
Calculating Technical Indicators
Technical indicators are mathematical calculations based on historical price, volume, or open interest information, and they help predict future price movements. We will calculate two simple indicators: Moving Average (MA) and Relative Strength Index (RSI).
Moving Average (MA)
A moving average smooths out price data by creating a constantly updated average price. The most common types are the Simple Moving Average (SMA) and the Exponential Moving Average (EMA).
# Simple Moving Average (SMA)
stock_data['SMA_20'] = stock_data['Adj Close'].rolling(window=20).mean()
# Exponential Moving Average (EMA)
stock_data['EMA_20'] = stock_data['Adj Close'].ewm(span=20, adjust=False).mean()
Relative Strength Index (RSI)
The RSI measures the speed and change of price movements, oscillating between 0 and 100, typically identifying overbought or oversold conditions.
def calculate_rsi(data, window):
delta = data['Adj Close'].diff(1)
gain = (delta.where(delta > 0, 0)).rolling(window=window).mean()
loss = (-delta.where(delta < 0, 0)).rolling(window=window).mean()
rs = gain / loss
rsi = 100 - (100 / (1 + rs))
return rsi
stock_data['RSI_14'] = calculate_rsi(stock_data, 14)
Developing a Basic Trading Algorithm
With our indicators in place, we can develop a simple trading algorithm. Let's create a basic strategy that buys a stock when its 20-day SMA is above the 50-day SMA and sells when it's below.
Define the Strategy
# Calculate longer-term SMA
stock_data['SMA_50'] = stock_data['Adj Close'].rolling(window=50).mean()
# Create buy/sell signals
stock_data['Signal'] = 0
stock_data['Signal'][20:] = np.where(stock_data['SMA_20'][20:] > stock_data['SMA_50'][20:], 1, 0)
stock_data['Position'] = stock_data['Signal'].diff()
In this code:
- We calculate the 50-day SMA.
- We generate buy/sell signals where a '1' signifies a buy signal and '0' signifies a sell signal.
- The
Position
column indicates changes in position based on the difference between consecutive signals.
Backtesting the Strategy
Backtesting involves testing the trading strategy on historical data to evaluate its performance.
initial_capital = 100000.0
stock_data['Holdings'] = stock_data['Adj Close'] * stock_data['Position'].cumsum()
stock_data['Cash'] = initial_capital - (stock_data['Adj Close'] * stock_data['Position']).cumsum()
stock_data['Total'] = stock_data['Cash'] + stock_data['Holdings']
# Calculate returns
stock_data['Returns'] = stock_data['Total'].pct_change()
# Print final portfolio value
print("Final Portfolio Value: ${}".format(stock_data['Total'].iloc[-1]))
Analyzing the Results
Analyzing the performance involves evaluating metrics such as cumulative returns, average returns, and volatility.
cumulative_returns = (stock_data['Total'].iloc[-1] - initial_capital) / initial_capital
average_daily_returns = stock_data['Returns'].mean()
volatility = stock_data['Returns'].std()
print("Cumulative Returns: {:.2f}%".format(cumulative_returns * 100))
print("Average Daily Returns: {:.4f}".format(average_daily_returns))
print("Volatility: {:.4f}".format(volatility))
These metrics provide a snapshot of the strategy's effectiveness and risk.
Further Learning
Developing trading algorithms is a continuous learning process. Here are some resources to deepen your understanding:
- "Python for Finance" by Yves Hilpisch: A comprehensive introduction to financial analytics and algorithmic trading using Python.
- QuantConnect: An algorithmic trading platform offering resources, tutorials, and a community of quants. It supports Python and provides historical data for backtesting.
- Kaggle: A platform for data science competitions offering datasets and notebooks related to financial markets and trading algorithms.
- Investopedia: A valuable resource for understanding financial concepts, including technical indicators and trading strategies.
- Coursera: Offers online courses on financial engineering, algorithmic trading, and Python programming for finance.
Conclusion
Developing basic trading algorithms using Python's pandas and NumPy libraries is a powerful way to harness data for informed trading decisions. This article provided a foundational guide to acquiring data, calculating technical indicators, implementing a simple trading strategy, and evaluating its performance. As you continue to explore and refine your algorithms, remember that financial markets are complex and ever-changing. Continuous learning and adaptation are key to success in algorithmic trading.