Automating Tasks with Python: A Complete Guide
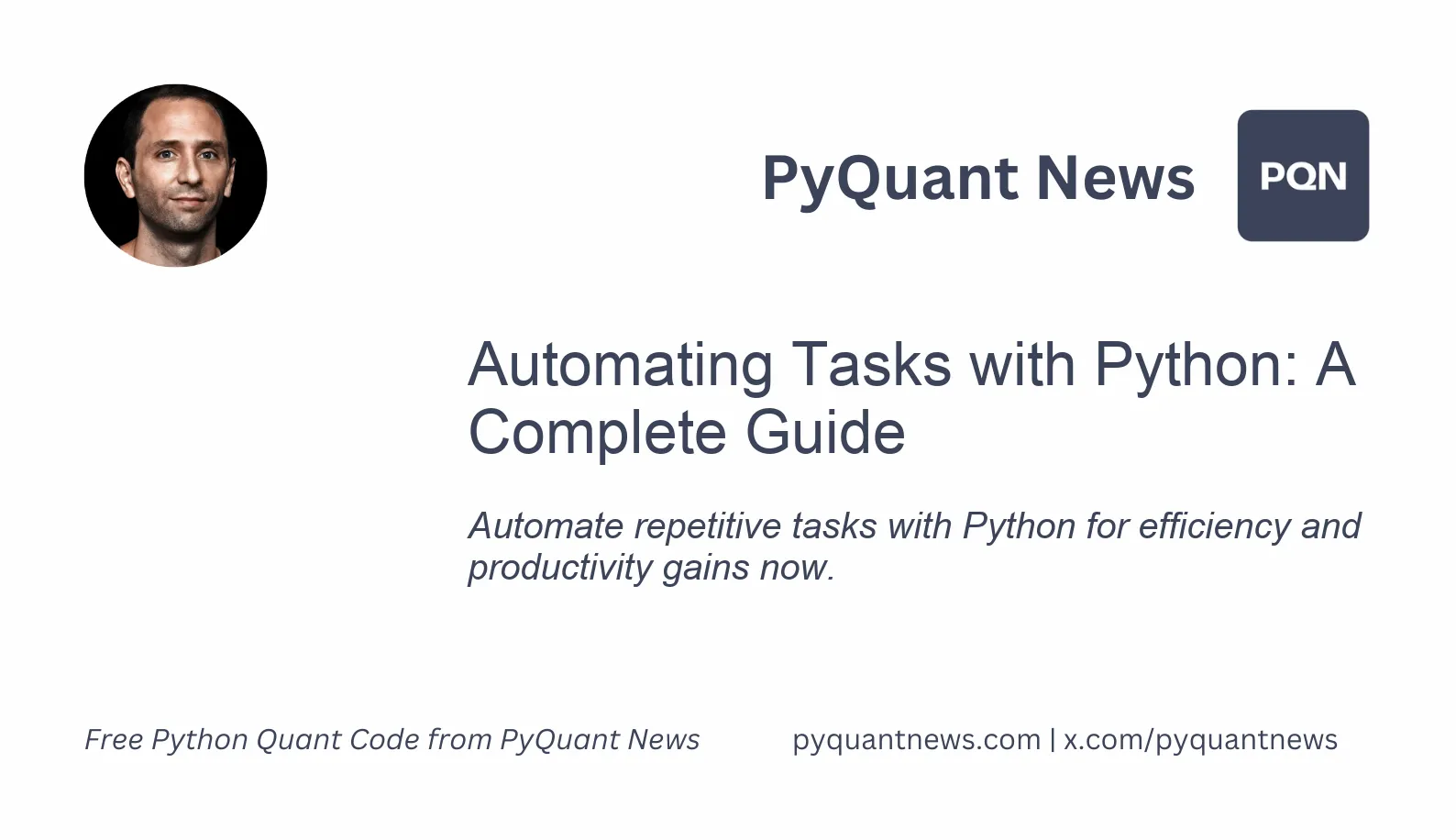
Automating Tasks with Python: A Complete Guide
Imagine a world where mundane tasks are handled seamlessly by a script running in the background, freeing you to focus on what really matters. Welcome to the power of Python automation. Whether you're an experienced coder optimizing your workflow, a busy professional seeking efficiency, or a novice eager to explore programming, this guide will show you how automating tasks with Python can transform repetitive chores into streamlined processes.
Why Use Python for Automation?
Python has become the go-to language for automation due to several key advantages:
- Ease of Learning: Python's syntax mirrors natural language, making it easy to read and write.
- Extensive Libraries: The Python ecosystem offers libraries for various automation needs—ranging from web scraping to file manipulation.
- Community Support: With a robust community, finding resources, tutorials, and help is easier than ever.
- Cross-Platform Compatibility: Python scripts run seamlessly on Windows, macOS, and Linux.
Getting Started with Python Automation
Before diving into automating tasks with Python, ensure you have Python installed on your machine. Download it from the official Python website. Consider creating a virtual environment to manage dependencies efficiently using python -m venv myenv
and activate it with source myenv/bin/activate
on macOS/Linux or myenv\Scripts\activate
on Windows.
Installing Essential Libraries
To automate tasks effectively, you'll need to install some essential libraries:
requests
: A simple library for making HTTP requests, perfect for web scraping and API interactions.beautifulsoup4
: For parsing HTML and XML documents.pandas
: For data manipulation and analysis.selenium
: For automating web browser interaction.
Install these libraries using pip:
pip install requests beautifulsoup4 pandas selenium
Writing Your First Automation Script
Let's begin with a simple example: downloading and saving images from a website.
Step 1: Import Libraries
import requests
from bs4 import BeautifulSoup
import os
Step 2: Fetch and Parse the Web Page
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
Step 3: Extract Image URLs
images = soup.find_all('img')
image_urls = [img['src'] for img in images]
Step 4: Download and Save Images
if not os.path.exists('images'):
os.makedirs('images')
for i, img_url in enumerate(image_urls):
img_data = requests.get(img_url).content
with open(f'images/image_{i}.jpg', 'wb') as handler:
handler.write(img_data)
Automating File Management
Managing files can be tedious, especially with large volumes of data. Python can automate tasks such as renaming files and organizing directories.
Renaming Files in Bulk
Consider a scenario where you need to rename multiple files in a directory by adding a prefix.
Step 1: Import Libraries
import os
Step 2: Define the Directory and Prefix
directory = '/path/to/your/files'
prefix = 'new_'
Step 3: Rename Files
for filename in os.listdir(directory):
old_path = os.path.join(directory, filename)
new_path = os.path.join(directory, prefix + filename)
os.rename(old_path, new_path)
Web Scraping for Data Collection
Web scraping is a powerful technique for collecting data from websites. Python makes web scraping easier than ever with its rich set of libraries. Always check a website’s robots.txt
file and terms of service to ensure you have permission to scrape their data.
Scraping Data from a Website
Let's scrape product information from an e-commerce website.
Step 1: Import Libraries
import requests
from bs4 import BeautifulSoup
import pandas as pd
Step 2: Fetch and Parse the Web Page
url = 'https://ecommerce-website.com/products'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
Step 3: Extract Product Information
products = []
for product in soup.find_all('div', class_='product'):
title = product.find('h2').text
price = product.find('span', class_='price').text
products.append({'Title': title, 'Price': price})
Step 4: Save Data to CSV
df = pd.DataFrame(products)
df.to_csv('products.csv', index=False)
Advanced Automation Techniques
As you become more comfortable with Python automation, you can explore more advanced techniques.
Automating Browser Tasks with Selenium
Selenium is a powerful tool for automating web browsers. It is particularly useful for tasks that require interaction with web pages, such as filling forms or clicking buttons.
Example: Automating Login to a Website
Step 1: Install WebDriver
Download the appropriate WebDriver for your browser from Selenium's official site.
Step 2: Import Libraries
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
Step 3: Launch Browser and Navigate to the Login Page
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('https://example.com/login')
Step 4: Locate and Fill Login Form
username = driver.find_element_by_name('username')
password = driver.find_element_by_name('password')
username.send_keys('your_username')
password.send_keys('your_password')
password.send_keys(Keys.RETURN)
Scheduling Tasks
You can automate the execution of your Python scripts using task schedulers. On Windows, you can use Task Scheduler, and on macOS or Linux, you can use cron jobs.
Example: Scheduling a Script to Run Daily
Step 1: Create a Python Script
Save your Python script (e.g., script.py
).
Step 2: Schedule the Script
Windows: Use Task Scheduler to create a new task and set the trigger to run daily.
macOS/Linux: Add a cron job by editing the crontab file:
crontab -e
Add the following line to run the script daily at 7 am:
0 7 * * * /usr/bin/python3 /path/to/script.py
Resources for Further Learning
Automation with Python is a vast and evolving field. To deepen your knowledge, consider exploring the following resources:
- Automate the Boring Stuff with Python by Al Sweigart: A comprehensive book that covers practical Python programming for beginners.
- Real Python: An excellent website offering tutorials, courses, and articles on Python programming.
- Python's Official Documentation: The official Python documentation is an invaluable resource for understanding Python's capabilities and libraries.
- Stack Overflow: A community-driven Q&A website where you can find answers to specific automation-related questions.
- GitHub: Explore open-source Python projects and repositories to see how others are using Python for automation.
Conclusion
Python automation has the potential to transform how you handle repetitive tasks. It makes your workflow more efficient and frees up time for more important activities. By leveraging Python's simplicity and powerful libraries, you can automate a wide range of tasks—from web scraping to file management and browser automation. As you continue to explore and experiment, you'll discover even more ways to harness the power of Python for automation.
Start small, build your skills, and soon you'll be a master of automating the mundane. This will unlock new levels of productivity and creativity.