Automate Trading with Interactive Brokers Python API
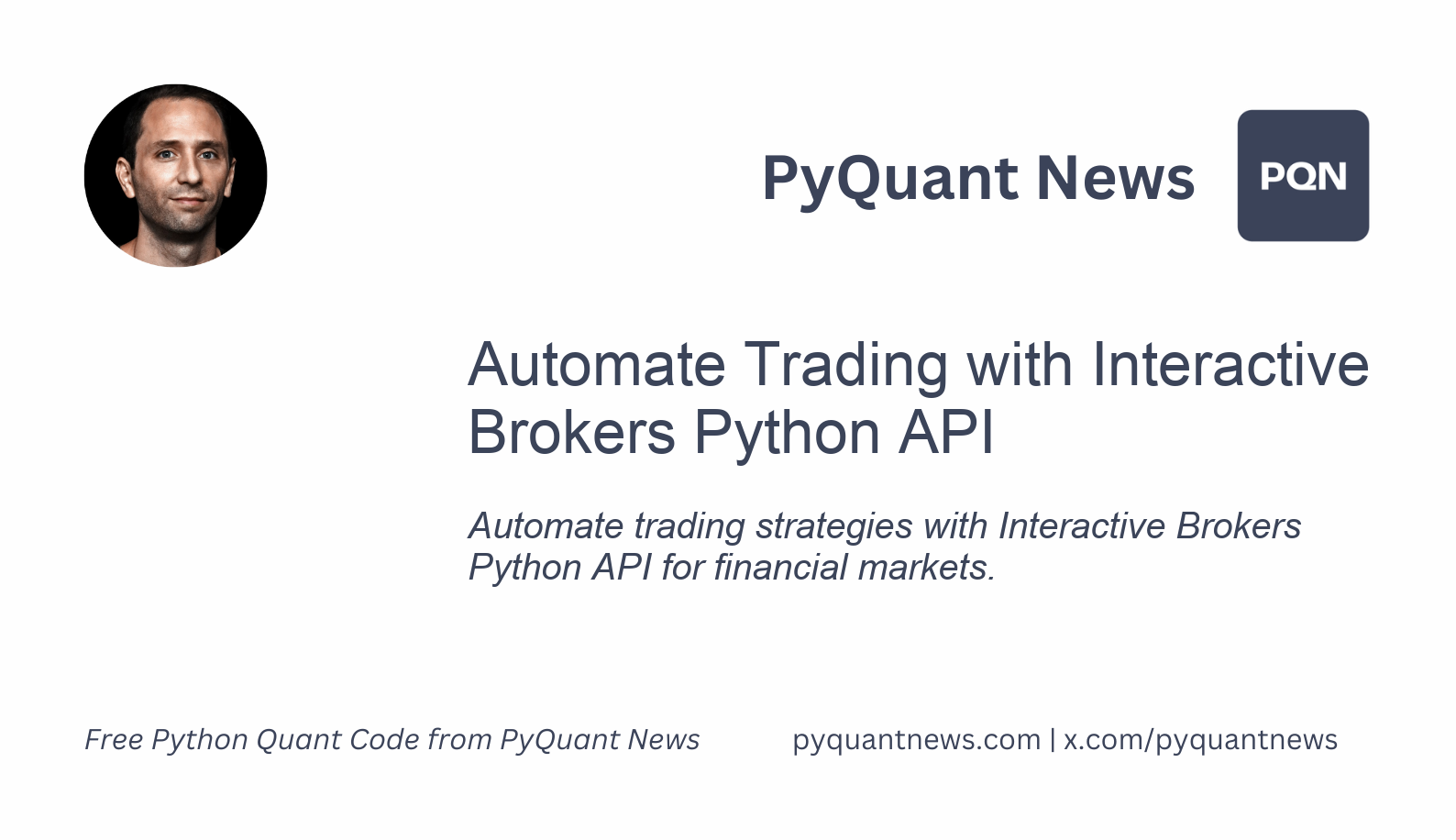
Automate Trading with Interactive Brokers Python API
In the fast-paced financial markets, automating trading strategies is a game-changer for traders seeking efficiency and enhanced performance. Interactive Brokers (IB) provides a powerful Python API that allows traders to automate their trading strategies seamlessly. This article delves into the Interactive Brokers Python API, its features, how to use it, and resources for mastering it.
Understanding the Interactive Brokers Python API
The Interactive Brokers Python API is a robust interface that lets traders interact programmatically with IB's trading platform. It offers access to market data, account information, and order management, making it ideal for developing and executing automated trading algorithms. Its extensive functionality appeals to both novice and experienced traders aiming to automate their strategies effectively.
Key Features
- Comprehensive Market Data Access: The API offers real-time and historical market data, essential for backtesting and live trading.
- Order Management: Place, modify, and cancel orders programmatically, ensuring precise control over trading strategies.
- Account and Portfolio Management: Access detailed account and portfolio information, enabling real-time monitoring of positions and performance.
- Event-Driven Programming: React to market changes with built-in event handling, executing trades based on predefined triggers.
Getting Started with the Interactive Brokers Python API
To begin automating trading strategies using the IB Python API, follow these steps to set up your environment and execute your first trade.
Setting Up the Environment
Before diving into coding, ensure you have an Interactive Brokers account and the necessary software and libraries:
- Interactive Brokers Account: Open an IB account if you don't have one, and enable API access in your settings.
- TWS or IB Gateway: Install the Trader Workstation (TWS) or IB Gateway to bridge your code with IB's servers.
- Python and
ibapi
: Install Python (preferably version 3.x) and theibapi
package, IB's official Python client library.
pip install ibapi
Establishing a Connection
To connect your Python script to the TWS or IB Gateway, use this basic example:
from ibapi.client import EClient
from ibapi.wrapper import EWrapper
class IBApi(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
def main():
app = IBApi()
app.connect("127.0.0.1", 7497, 0)
app.run()
if __name__ == "__main__":
main()
This script sets up a connection to the TWS or IB Gateway running on your local machine. Adjust the connection parameters as needed.
Fetching Market Data
Accessing real-time market data is crucial for informed trading decisions. Here's how to request data for a specific stock:
from ibapi.contract import Contract
class IBApi(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
def nextValidId(self, orderId):
self.start()
def start(self):
contract = Contract()
contract.symbol = "AAPL"
contract.secType = "STK"
contract.exchange = "SMART"
contract.currency = "USD"
self.reqMarketDataType(1)
self.reqMktData(1, contract, "", False, False, [])
def tickPrice(self, reqId, tickType, price, attrib):
print(f"Tick Price. Ticker Id: {{reqId}}, tickType: {{tickType}}, Price: {{price}}")
def main():
app = IBApi()
app.connect("127.0.0.1", 7497, 0)
app.run()
if __name__ == "__main__":
main()
This script defines a contract for Apple Inc. (AAPL) and requests real-time market data. The tickPrice
method handles price updates and prints them to the console.
Placing Orders
Automate trades by placing orders programmatically. Here's how to place a market order:
from ibapi.order import Order
class IBApi(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
def nextValidId(self, orderId):
self.nextOrderId = orderId
self.start()
def start(self):
contract = Contract()
contract.symbol = "AAPL"
contract.secType = "STK"
contract.exchange = "SMART"
contract.currency = "USD"
order = Order()
order.action = "BUY"
order.orderType = "MKT"
order.totalQuantity = 10
self.placeOrder(self.nextOrderId, contract, order)
def main():
app = IBApi()
app.connect("127.0.0.1", 7497, 0)
app.run()
if __name__ == "__main__":
main()
This example places a market order to buy 10 shares of AAPL. The nextValidId
method ensures a unique order ID for each trade.
Advanced Automation Techniques
With the basics covered, explore advanced automation techniques like algorithmic trading and backtesting.
Algorithmic Trading
Algorithmic trading uses predefined rules and mathematical models to execute trades. Develop algorithms based on technical indicators, statistical models, or machine learning techniques. The IB Python API supports various strategies, including:
- Mean Reversion: Buying and selling assets based on their deviation from historical averages.
- Momentum Trading: Capitalizing on trends by buying rising assets and selling falling ones.
- Arbitrage: Exploiting price discrepancies between markets or instruments.
Backtesting
Backtesting tests a strategy against historical data to evaluate performance. The IB Python API lets you fetch historical data and simulate trades, providing insights into a strategy's viability.
def fetch_historical_data(contract, duration, bar_size):
app.reqHistoricalData(1, contract, "", duration, bar_size, "MIDPOINT", 1, 1, False, [])
class IBApi(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
def nextValidId(self, orderId):
self.start()
def start(self):
contract = Contract()
contract.symbol = "AAPL"
contract.secType = "STK"
contract.exchange = "SMART"
contract.currency = "USD"
fetch_historical_data(contract, "1 Y", "1 day")
def historicalData(self, reqId, bar):
print(f"Date: {{bar.date}}, Close: {{bar.close}}")
def main():
app = IBApi()
app.connect("127.0.0.1", 7497, 0)
app.run()
if __name__ == "__main__":
main()
This script fetches one year of daily historical data for AAPL and prints the closing prices. Use this data to backtest and refine your trading strategies.
Resources for Learning More
To further your knowledge of the Interactive Brokers Python API and improve your trading automation skills, explore these resources:
- Interactive Brokers API Documentation: The official documentation provides comprehensive details on API functions, parameters, and usage examples. Visit Interactive Brokers API Guide for more information.
- YouTube Tutorials: Channels like "QuantInsti" and "Trading With Python" offer step-by-step guides and practical examples of using the IB Python API.
- Online Courses: Platforms like Udemy and Coursera provide courses on algorithmic trading and API integration. Consider courses such as "Algorithmic Trading with Interactive Brokers API" to build a strong foundation.
- Forums and Communities: Engage with online communities like the IB API Forum and Stack Overflow for valuable insights and solutions to common challenges.
- Books: "Python for Algorithmic Trading" by Yves Hilpisch offers in-depth knowledge of using Python for trading automation, including practical examples with the IB API.
Conclusion
The Interactive Brokers Python API opens up numerous possibilities for traders looking to automate their strategies. Leveraging its comprehensive features and capabilities can enhance efficiency and optimize trading strategies, helping traders stay ahead in the competitive financial markets. With the right resources and dedication to learning, mastering the IB Python API can be a rewarding journey, enabling traders to achieve their financial goals with precision and confidence.
Disclaimer: The information provided in this article is for educational purposes only and does not constitute financial advice. Trading involves risk, and individuals should conduct thorough research and consult with a financial advisor before making any trading decisions.