When I turned 18, I made two choices that transformed my life:
First, I learned how to code to build my own analytics apps.
Second, I traded my first stock.
The first code I wrote was PHP and it was terrible.
The first stock I traded was MSFT and I lost money.
Since then, I’ve written hundreds of thousands of code lines and traded thousands of stocks.
Most of the code I’ve written is to do one thing:
And most of the time, I do it in a web app (see here and here).
The problem is, it can take days (or years) to get all the parts of the web app right.
Nailing the layout, making it work, and organizing the files just right is pretty time-consuming.
Fortunately, I discovered Streamlit when it launched in 2018 and it’s a game-changer.
Now I build analytics web apps in 5 minutes.
And you can too.
Build your own market data analytics app in 5 minutes
Building Streamlit web apps lets you showcase your skills to employers by Building in Public.
Startups, bootstrappers, indie software developers, and of course Python developers Build in Public to showcase their skills, get help, and establish their networks.
You can also quickly build reusable analytics in a web browser. This makes it easy to include analytics in your investment workflow.
Let’s dive in.
Imports and set up
Streamlit is a Python library that lets you create web apps easily. Today, you will use pandas
and yfinance
to download and process the stock data, and the talib
library for technical analysis.
Create a new Python script called stock_data_analysis.py
, and import the necessary libraries.
import streamlit as st import pandas as pd import yfinance as yf import talib You can find instructions for installing TA-Lib here.
Create the app
Your app will let people choose a ticker from the S&P 500 index. Create a function to download the symbols from Wikipedia.
def get_sp500_components(): df = pd.read_html("<a href="https://en.wikipedia.org/wiki/List_of_S%26P_500_companies" target="_blank" rel="noreferrer noopener">https://en.wikipedia.org/wiki/List_of_S%26P_500_companies</a>") df = df[0] tickers = df["Symbol"].to_list() tickers_companies_dict = dict(zip(df["Symbol"], df["Security"])) return tickers, tickers_companies_dict
Then create a function that applies a technical analysis indicator depending on the selection.
indicators = [<br> "Simple Moving Average",<br> "Exponential Moving Average",<br> "Relative Strength Index",<br>]<br><br>def apply_indicator(indicator, data):<br> if indicator == "Simple Moving Average":<br> sma = talib.SMA(data["Close"])<br> return pd.DataFrame({"Close": data["Close"], "SMA": sma})<br> elif indicator == "Exponential Moving Average":<br> ema = talib.EMA(data["Close"])<br> return pd.DataFrame({"Close": data["Close"], "EMA": ema})<br> elif indicator == "Relative Strength Index":<br> rsi = talib.RSI(data["Close"])<br> return pd.DataFrame({"Close": data["Close"], "RSI": rsi})<br>
Set the title of the app, add a brief description, and set up the select boxes.
st.title("Stock Data Analysis") st.write("A simple app to download stock data and apply technical analysis indicators.") st.sidebar.header("Stock Parameters") available_tickers, tickers_companies_dict = get_sp500_components() ticker = st.sidebar.selectbox( "Ticker", available_tickers, format_func=tickers_companies_dict.get ) start = st.sidebar.date_input("Start date:", pd.Timestamp("2020-01-01")) end = st.sidebar.date_input("End date:", pd.Timestamp("2021-12-31"))
Finally, download the data, apply the selected indicator, and create the chart and data table.
data = yf.download(ticker, start, end) selected_indicator = st.selectbox("Select a technical analysis indicator:", indicators) indicator_data = apply_indicator(selected_indicator, data) st.write(f"{selected_indicator} for {ticker}") st.line_chart(indicator_data) st.write("Stock data for", ticker) st.dataframe(data)
That’s it! Now to run the app, enter the command at your terminal window in the same directory as the script you created.
streamlit run stock_data_analysis.py
In a few seconds, your browser window will open and your app will launch!
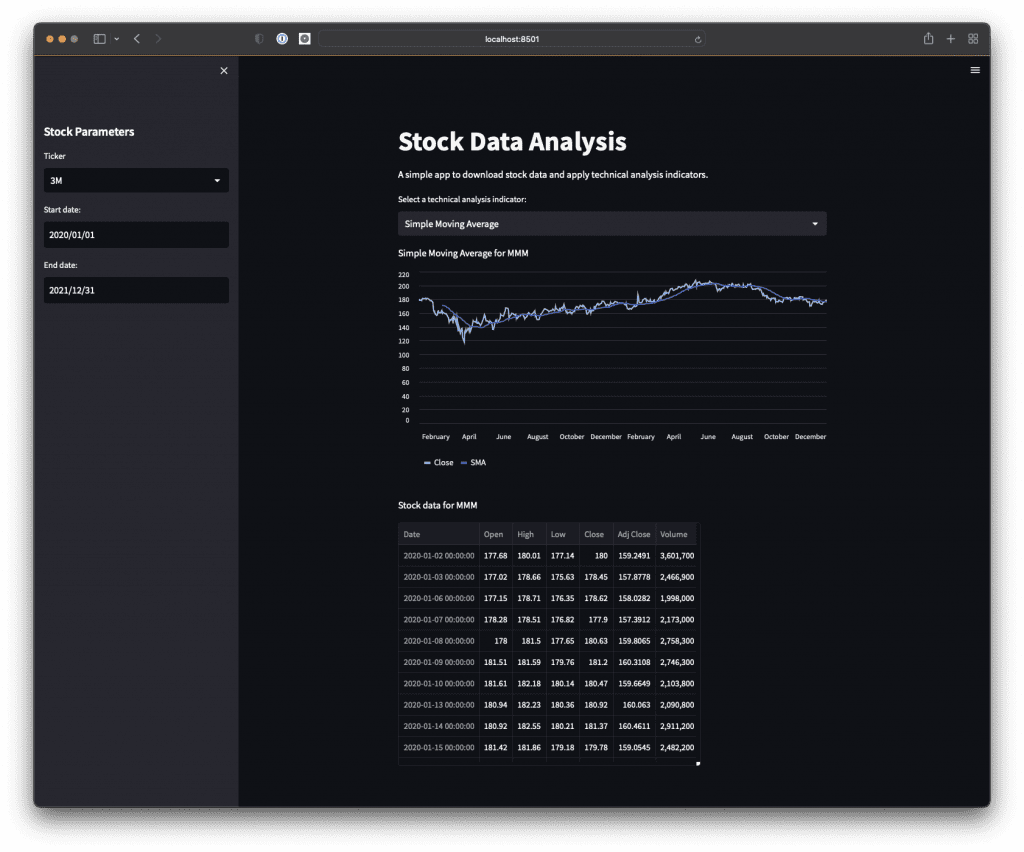
This app only touches on what you can do with Streamlit. Incorporate other technical analyses, download economic or other market data, and even host your app for others to use. Now you have an app to build on and make it yours.
And it only took 5 minutes.