1.4 million options contracts: Stream it all real-time with ThetaData

1.4 million options contracts: Stream it all real-time with ThetaData
I’ve been trading options contracts for more than 23 years.
When I started out, I had to rely on expensive broker data feeds for real-time data for trading and low-quality free data I scraped from websites for analysis.
I spent countless hours reverse engineering the CBOE website for live data, only to have my IP address blocked.
I spent $1,125 on 5 years of historic options data for one symbol (RUT) from iVolatility but that’s only a snapshot (and super expensive).
I even took 20-minute delayed snapshots from Yahoo and treated it as real-time, only to consistently lose money.
Things have improved for people like us, but not by much.
Free data is still delayed, filtered, and low quality.
Real-time streaming data is generally available on broker platforms for trading, but unavailable for analysis.
Until now.
1.4 million active options contracts: Real-time, streaming with ThetaData
The Options Price Reporting Authority (OPRA) is a securities information processor that consolidates options quotes and trade data from major US exchanges.
There are 1.4 million active options contracts trading at any time which generate over 3 terabytes of data each day.
OPRA consolidates and publishes these data in real-time.
Disseminating these data, unfiltered, to non-professionals has been made available through ThetaData, which has a connection to OPRA.
The ThetaData Python API streams quotes and trades in milliseconds because it’s compressed to 1/30th of the actual size.
And the best part? A ThetaData subscription is dirt cheap.
Let’s see how it works.
Imports and set up
Start by importing the ThetaData client classes. (You’ll need Java installed, instructions here.)
from datetime import date
1from datetime import date
2
3import thetadata.client
4from thetadata import (
5 Quote,
6 StreamMsg,
7 ThetaClient,
8 OptionRight,
9 StreamMsgType
10)
11from thetadata import StreamResponseType as ResponseType
Then create a few variables you will use to grab the data.
1last_call_quote = Quote()
2last_put_quote = Quote()
3price = 0
Create a callback
A callback is a function that is called as a result of an event. ThetaData uses callbacks to do something in response to a quote or trade.
1def callback_straddle(msg):
2
3 if (msg.type != StreamMsgType.QUOTE):
4 return
5
6 global price
7
8 if msg.contract.isCall:
9 last_call_quote.copy_from(msg.quote)
10 else:
11 last_put_quote.copy_from(msg.quote)
12
13 straddle_bid = round(last_call_quote.bid_price + last_put_quote.bid_price, 2)
14 straddle_ask = round(last_call_quote.ask_price + last_put_quote.ask_price, 2)
15 straddle_mid = round((straddle_bid + straddle_ask) / 2, 2)
16
17 time_stamp = thetadata.client.ms_to_time(
18 msg.quote.ms_of_day
19 )
20
21 if price != straddle_mid:
22 print(
23 f"time: {time_stamp} bid: {straddle_bid} mid: {straddle_mid} ask: {straddle_ask}"
24 )
25 price = straddle_mid
In this function, you’ll first make sure the message type is a quote. If it’s not, return and do nothing. Otherwise, set the quotes for the call and put options you use to create your straddle. Then, calculate the bid and ask prices of the straddle and divide by two to get the midpoint. Finally, record the quote timestamp and print the result.
Connect and stream the data
Next, connect to ThetaData’s servers, start the stream for the call an put, and check the subscription status.
1def streaming_straddle():
2
3 client = ThetaClient(
4 username="",
5 passwd=""
6 )
7
8 client.connect_stream(
9 callback_straddle
10 )
11
12 req_id_call = client.req_quote_stream_opt(
13 "SPY", date(2023, 5, 5), 410, OptionRight.CALL
14 ) # Request quote updates
15
16 req_id_put = client.req_quote_stream_opt(
17 "SPY", date(2023, 5, 5), 410, OptionRight.PUT
18 )
19
20 if (
21 client.verify(req_id_call) != ResponseType.SUBSCRIBED
22 or client.verify(req_id_put) != ResponseType.SUBSCRIBED
23 ):
24 raise Exception(
25 "Unable to stream contract. A standard/PRO subscription required."
26 )
This code sets the callback to respond to every quote update for the 5 May 2023 SPY options. You can use any symbol and expiration you want.
To start the stream, just call the function you just created.
1streaming_straddle()
You’ll start to see data print to the screen as new quotes are received.
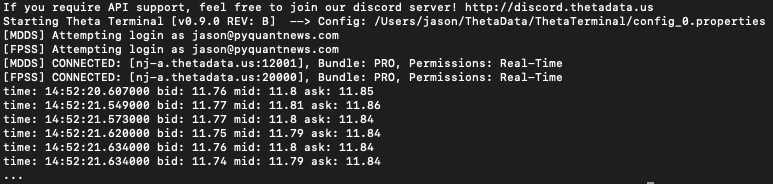
ThetaData’s API provides first, second, and third-order Greeks, implied volatility, volume, open interest, and more.
With ThetaData, you have the same tools the professionals have.
